ralke
(҂ ͠❛ ෴ ͡❛)ᕤ
- Joined
- Dec 17, 2011
- Messages
- 1,714
- Solutions
- 31
- Reaction score
- 967
- Location
- Santiago - Chile
- GitHub
- ralke23
- Twitch
- ralke23
Hi there guys! I don't know where to ask for help with this. I'm struggling with Unity, doing a player movement script.
What I need is to add a bool for every time that the player turns.
I have this
And I would like to change it this way:
And this for the 8 directions (adding north-east, south-west, etc.) How can I merge this into the code above? I know the player can rotate withouth having more animations than 1 .fbx, but I really need to load the 8 direction animations separately.
Also I know that h, and v can't access to 45º rotations, for this I though on
answers.unity.com
This way I could use:
And load the player gameObject as public variable, but I have no idea how could I merge this with the code above to work properly
The outcome I need to achieve is to import my character animations on .fbx with baked isometry for each direction...
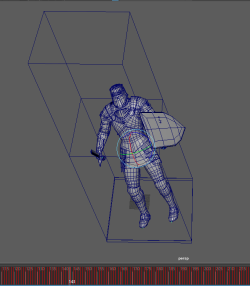
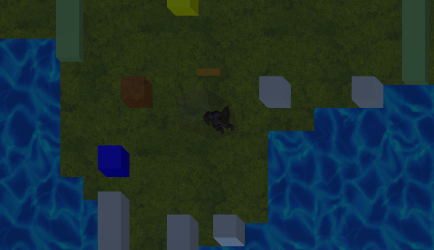
But it breaks when I rotate
Thanks in advance!
Regards
What I need is to add a bool for every time that the player turns.
I have this
C#:
void Update()
{
// Get player data (input)
float h = Input.GetAxisRaw("Horizontal");
float v = Input.GetAxisRaw("Vertical");
// Save in xyz
Vector3 direction = new Vector3(h,0,v);
// Apply the movement
// Use transform.Position(); if character teleports
transform.Translate(direction * playerSpeed * Time.deltaTime, Space.World);
// If player moves, turn direction
if (direction != Vector3.zero)
{
// Generate advanced rotation, rotate where you look
Quaternion rota = Quaternion.LookRotation(direction, Vector3.up);
// Apply rotations to character
transform.rotation = Quaternion.RotateTowards(transform.rotation, rota, playerTurn);
// Text variable (string) that calls Animator's boolean
playerAnimator.SetBool("Caminar", true);
}
And I would like to change it this way:
C#:
if h = -1 ///(this mean is looking to left),
{ playerAnimator.SetBool("Walk_Left", true; }
if h = 1 // this mean is looking right
{ playerAnimator.SetBool("Walk_Right", true; }
And this for the 8 directions (adding north-east, south-west, etc.) How can I merge this into the code above? I know the player can rotate withouth having more animations than 1 .fbx, but I really need to load the 8 direction animations separately.
Also I know that h, and v can't access to 45º rotations, for this I though on
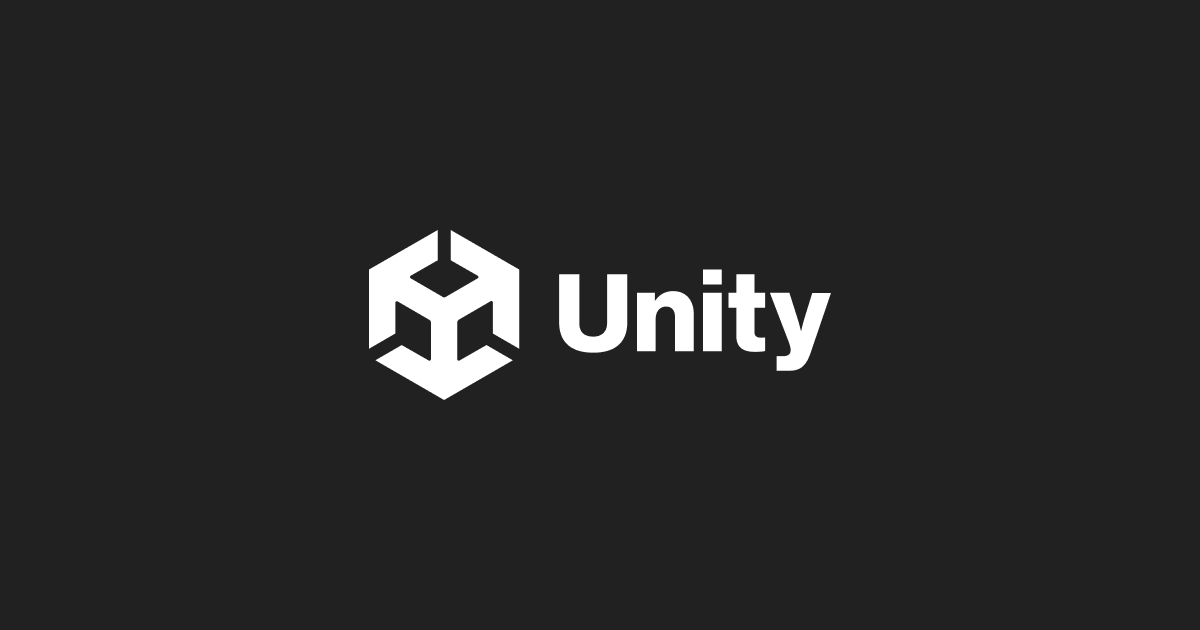
Check an Objects Rotation
Hello, I know there is a way to check the position of an object along x, y and z, but is it possible to check an objects rotation along x, y and z also? My intention for this is to rotate a cube by 90 degrees along the y axis and to check its rotation using an if statement to trigger an event...

This way I could use:
C#:
if (myObject.transform.rotation.eulerAngles.y == 45)
{
playerAnimator.SetBool("Walk_NorthWest", true);
}
C#:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour
{
// Local variables
public float playerSpeed;
public float playerTurn;
public GameObject ballTrigger;
// Modifify Animator parameters
public Animator playerAnimator;
private Vector3 lastMove;
void Start()
{
// Access to Animator component on start
playerAnimator = GetComponent<Animator>();
ballTrigger.gameObject.SetActive(false);
playerSpeed = 1;
}
void Update()
{
// Get player data (input)
float h = Input.GetAxisRaw("Horizontal");
float v = Input.GetAxisRaw("Vertical");
// Save in xyz
Vector3 direction = new Vector3(h,0,v);
// Apply the movement
// Use transform.Position(); if character teleports
transform.Translate(direction * playerSpeed * Time.deltaTime, Space.World);
// If player moves, turn direction
if (direction != Vector3.zero)
{
// Generate advanced rotation, rotate where you look
Quaternion rota = Quaternion.LookRotation(direction, Vector3.up);
// Apply rotations to character
transform.rotation = Quaternion.RotateTowards(transform.rotation, rota, playerTurn);
// Text variable (string) that calls Animator's boolean
playerAnimator.SetBool("Caminar", true);
}
else if (Input.GetKey(KeyCode.E))
{
Debug.Log("Using E");
// Text variable (string) that calls Animator's boolean
// Apply rotations to character
playerAnimator.SetBool("Shield", true);
playerAnimator.SetBool("Caminar", false);
ballTrigger.gameObject.SetActive(true);
playerSpeed = 0;
}
else if (Input.GetAxisRaw("Horizontal") > 0.5f || Input.GetAxisRaw("Horizontal") < -0.5f)
{
transform.Translate(new Vector3(Input.GetAxisRaw("Horizontal") * playerSpeed * Time.deltaTime, 0f, 0f));
lastMove = new Vector3(Input.GetAxisRaw("Horizontal"), 0f);
}
else
{
playerAnimator.SetBool("Caminar", false);
playerAnimator.SetBool("Shield", false);
ballTrigger.gameObject.SetActive(false);
playerSpeed = 3;
}
}
}
The outcome I need to achieve is to import my character animations on .fbx with baked isometry for each direction...
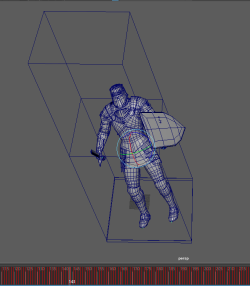
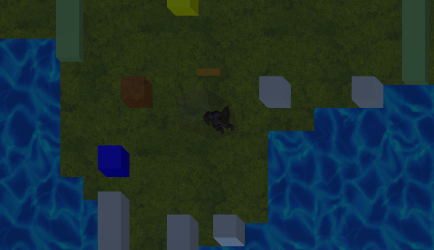
But it breaks when I rotate
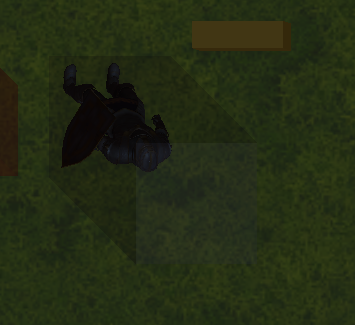
Thanks in advance!
Regards
Last edited: