Vivelo
New Member
- Joined
- Aug 30, 2018
- Messages
- 34
- Reaction score
- 3
Hello everybody,
as in the topic, I am looking for a script that will bring up the stats of an item when hovering over it with the mouse.
I'm not very good at programming but maybe some of you can help me.
Perhaps the script inventory.lua in which my character's outfit is displayed will help?
Here is a graphic example:
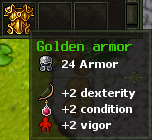
THANKS FOR ALL HELP!
Edit: I need script for TFS 0.3.6
as in the topic, I am looking for a script that will bring up the stats of an item when hovering over it with the mouse.
I'm not very good at programming but maybe some of you can help me.
Perhaps the script inventory.lua in which my character's outfit is displayed will help?
Lua:
InventorySlotStyles = {
[InventorySlotHead] = "HeadSlot",
[InventorySlotNeck] = "NeckSlot",
[InventorySlotBack] = "BackSlot",
[InventorySlotBody] = "BodySlot",
[InventorySlotRight] = "RightSlot",
[InventorySlotLeft] = "LeftSlot",
[InventorySlotLeg] = "LegSlot",
[InventorySlotFeet] = "FeetSlot",
[InventorySlotFinger] = "FingerSlot",
[InventorySlotAmmo] = "AmmoSlot",
[InventorySlotOutfit] = "OutfitSlot"
}
outfitinv = nil
inventoryWindow = nil
inventoryPanel = nil
inventoryButton = nil
purseButton = nil
healthInfoWindow = nil
healthBar = nil
manaBar = nil
experienceBar = nil
soulLabel = nil
capLabel = nil
healthTooltip = "Your character health is %d out of %d."
manaTooltip = "Your character mana is %d out of %d."
experienceTooltip = "You have %d%% to advance to level %d."
function init()
connect(
LocalPlayer,
{
onInventoryChange = onInventoryChange,
onBlessingsChange = onBlessingsChange
}
)
connect(g_game, {onGameStart = refresh})
g_keyboard.bindKeyDown("Ctrl+I", toggle)
inventoryButton = modules.client_topmenu.addRightGameToggleButton("inventoryButton", tr("Inventory") .. " (Ctrl+I)", "/images/topbuttons/inventory", toggle)
inventoryButton:setOn(true)
inventoryWindow = g_ui.loadUI("inventory", modules.game_interface.getRightPanel())
inventoryWindow:disableResize()
inventoryPanel = inventoryWindow:getChildById("contentsPanel")
purseButton = inventoryPanel:getChildById("purseButton")
local function purseFunction()
local purse = g_game.getLocalPlayer():getInventoryItem(InventorySlotPurse)
if purse then
g_game.use(purse)
end
end
purseButton.onClick = purseFunction
refresh()
inventoryWindow:setup()
end
function terminate()
disconnect(
g_game,
{
onOpenOutfitWindow = create,
onGameEnd = destroy
}
)
destroy()
end
function terminate()
disconnect(
LocalPlayer,
{
onInventoryChange = onInventoryChange,
onBlessingsChange = onBlessingsChange
}
)
disconnect(g_game, {onGameStart = refresh})
g_keyboard.unbindKeyDown("Ctrl+I")
inventoryWindow:destroy()
inventoryButton:destroy()
end
function toggleAdventurerStyle(hasBlessing)
for slot = InventorySlotFirst, InventorySlotLast do
local itemWidget = inventoryPanel:getChildById("slot" .. slot)
if itemWidget then
itemWidget:setOn(hasBlessing)
end
end
end
function refresh()
local player = g_game.getLocalPlayer()
for i = InventorySlotFirst, InventorySlotPurse do
if g_game.isOnline() then
onInventoryChange(player, i, player:getInventoryItem(i))
else
onInventoryChange(player, i, nil)
end
toggleAdventurerStyle(player and Bit.hasBit(player:getBlessings(), Blessings.Adventurer) or false)
end
local creatureBox = inventoryPanel:getChildById("slot12")
local player = g_game.getLocalPlayer()
if player then
creatureBox:setCreature(player)
end
purseButton:setVisible(g_game.getFeature(GamePurseSlot))
end
function toggle()
if inventoryButton:isOn() then
inventoryWindow:close()
inventoryButton:setOn(false)
else
inventoryWindow:open()
inventoryButton:setOn(true)
end
end
function onMiniWindowClose()
inventoryButton:setOn(false)
end
-- hooked events
function onInventoryChange(player, slot, item, oldItem)
if slot > InventorySlotPurse then
return
end
if slot == InventorySlotPurse then
if g_game.getFeature(GamePurseSlot) then
purseButton:setEnabled(item and true or false)
end
return
end
local itemWidget = inventoryPanel:getChildById("slot" .. slot)
if item then
itemWidget:setStyle("InventoryItem")
itemWidget:setItem(item)
else
itemWidget:setStyle(InventorySlotStyles[slot])
itemWidget:setItem(nil)
end
end
function onBlessingsChange(player, blessings, oldBlessings)
local hasAdventurerBlessing = Bit.hasBit(blessings, Blessings.Adventurer)
if hasAdventurerBlessing ~= Bit.hasBit(oldBlessings, Blessings.Adventurer) then
toggleAdventurerStyle(hasAdventurerBlessing)
end
end
Here is a graphic example:
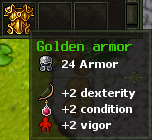
THANKS FOR ALL HELP!
Edit: I need script for TFS 0.3.6
Last edited: