Hello there, i just want to release an small tutorial how to check an item on specific position. It can be on a tile, an board or what ever.
In this small tutorial we will make an tile, and an lever to pull. When you pull the lever it should check if the item is on the tile.
Lets start!
STEP 1
Try to build an area like this :
Sorry for this noob pic ;p
Lets make an new file, call it position.lua ..
Lets open it, and now we will make an small config to check what item and what you recive so start like :
This is how to start the config, now we will fill it with some information, start like this:
STEP 2
Now we will have the configuration, no we will continue with the functions. Start like this :
Now we have added the function onUse and the position of the specific tile to check.
Now continue with :
Now we have added it to check for the item, and if it remove the item
Now we continue with the switch ID's, do something like this :
Now we added basic to check the lever id's so if goes right and left and execute the scripts from both ways.
Now we will add so it gives you the item if the item are right.
Continue with this:
Now you have added so the player get the item, now we will continue with the msg if the item are wrong
Continue with this:
Now you are done with the script! Now we need to add this script and put right unique id on switch.
On the switch, put unique id "41242" in mapeditor.
And now open actions.xml and add this line :
Now you are done, and the script should look like this :
I hope this will help you understand some functions in LUA scripting!
Enjoy
In this small tutorial we will make an tile, and an lever to pull. When you pull the lever it should check if the item is on the tile.
Lets start!
STEP 1
Try to build an area like this :
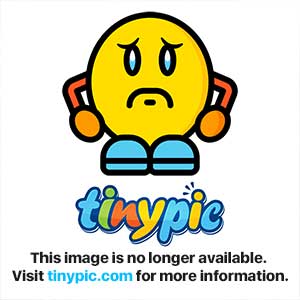
Sorry for this noob pic ;p
Lets make an new file, call it position.lua ..
Lets open it, and now we will make an small config to check what item and what you recive so start like :
Lua:
local config = {
--- Here we will put the config in next step ---
}
This is how to start the config, now we will fill it with some information, start like this:
Lua:
local config = {
item = 8930, --- The item you need to put on the specific tile ---
toget = 2160, --- The item you will get when you pull the lever and remove the item ---
}
STEP 2
Now we will have the configuration, no we will continue with the functions. Start like this :
Lua:
local config = {
item = 8930, --- The item you need to put on the specific tile ---
toget = 2160, --- The item you will get when you pull the lever and remove the item ---
}
function onUse(cid, item, fromPosition, itemEx, toPosition) --- This always added if you gonna use something ---
local pos = {x = 1000, y = 1000, z = 7} --- This is the position left from the switch ---
Now we have added the function onUse and the position of the specific tile to check.
Now continue with :
Lua:
local config = {
item = 8930, --- The item you need to put on the specific tile ---
toget = 2160, --- The item you will get when you pull the lever and remove the item ---
}
function onUse(cid, item, fromPosition, itemEx, toPosition) --- This always added if you gonna use something ---
local pos = {x = 1000, y = 1000, z = 7} --- This is the position left from the switch, this is called "pos"---
if getTileItemById(pos, config.item).uid > 0 then --- Here it check after the item ---
if doRemoveItem(getTileItemById(pos, config.item).uid,1) then --- Here if the item is right then it remove it ---
Now we have added it to check for the item, and if it remove the item
Now we continue with the switch ID's, do something like this :
Lua:
local config = {
item = 8930, --- The item you need to put on the specific tile ---
toget = 2160, --- The item you will get when you pull the lever and remove the item ---
}
function onUse(cid, item, fromPosition, itemEx, toPosition) --- This always added if you gonna use something ---
local pos = {x = 1000, y = 1000, z = 7} --- This is the position left from the switch, this is called "pos"---
if getTileItemById(pos, config.item).uid > 0 then --- Here it check after the item ---
if doRemoveItem(getTileItemById(pos, config.item).uid,1) then --- Here if the item is right then it remove it ---
--- Switch id's ---
if item.itemid == 1945 then
doTransformItem(item.uid, 1946)
elseif item.itemid == 1946 then
doTransformItem(item.uid, 1945)
end
--- Switch id's ---
Now we added basic to check the lever id's so if goes right and left and execute the scripts from both ways.
Now we will add so it gives you the item if the item are right.
Continue with this:
Lua:
local config = {
item = 8930, --- The item you need to put on the specific tile ---
toget = 2160, --- The item you will get when you pull the lever and remove the item ---
}
function onUse(cid, item, fromPosition, itemEx, toPosition) --- This always added if you gonna use something ---
local pos = {x = 1000, y = 1000, z = 7} --- This is the position left from the switch, this is called "pos"---
if getTileItemById(pos, config.item).uid > 0 then --- Here it check after the item ---
if doRemoveItem(getTileItemById(pos, config.item).uid,1) then --- Here if the item is right then it remove it ---
--- Switch id's ---
if item.itemid == 1945 then
doTransformItem(item.uid, 1946)
elseif item.itemid == 1946 then
doTransformItem(item.uid, 1945)
end
--- Switch id's ---
doPlayerAddItem(cid, config.toget) --- If the item is right and on right tile "pos" then it execute this ---
--- Add this bit to, to close the script ---
return true
end
end
--- Add this bit to, to close the script ---
Now you have added so the player get the item, now we will continue with the msg if the item are wrong
Continue with this:
Lua:
local config = {
item = 8930, --- The item you need to put on the specific tile ---
toget = 2160, --- The item you will get when you pull the lever and remove the item ---
}
function onUse(cid, item, fromPosition, itemEx, toPosition) --- This always added if you gonna use something ---
local pos = {x = 1000, y = 1000, z = 7} --- This is the position left from the switch, this is called "pos"---
if getTileItemById(pos, config.item).uid > 0 then --- Here it check after the item ---
if doRemoveItem(getTileItemById(pos, config.item).uid,1) then --- Here if the item is right then it remove it ---
--- Switch id's ---
if item.itemid == 1945 then
doTransformItem(item.uid, 1946)
elseif item.itemid == 1946 then
doTransformItem(item.uid, 1945)
end
--- Switch id's ---
doPlayerAddItem(cid, config.toget) --- If the item is right and on right tile "pos" then it execute this ---
--- Add this bit to, to close the script ---
return true
end
end
--- Add this bit to, to close the script ---
--- If the item is wrong, it shows this message ---
doPlayerSendTextMessage(cid, MESSAGE_EVENT_ORANGE, "You dont have put the right items on the table!")
return true
end
--- If the item is wrong, it shows this message ---
Now you are done with the script! Now we need to add this script and put right unique id on switch.
On the switch, put unique id "41242" in mapeditor.
And now open actions.xml and add this line :
Lua:
<action uniqueid="41242" event="script" value="position.lua"/>
Now you are done, and the script should look like this :
Lua:
local config = {
item = 8930,
toget = 2160,
}
function onUse(cid, item, fromPosition, itemEx, toPosition)
local pos = {x = 1000, y = 1000, z = 7}
if getTileItemById(pos, config.item).uid > 0 then
if doRemoveItem(getTileItemById(pos, config.item).uid,1) then
if item.itemid == 1945 then
doTransformItem(item.uid, 1946)
elseif item.itemid == 1946 then
doTransformItem(item.uid, 1945)
end
doPlayerAddItem(cid, config.toget)
return true
end
end
doPlayerSendTextMessage(cid, MESSAGE_EVENT_ORANGE, "You dont have put the right items on the table!")
return true
end
I hope this will help you understand some functions in LUA scripting!
Enjoy