Zisly
Intermediate OT User
- Joined
- Jun 9, 2008
- Messages
- 7,338
- Reaction score
- 120
I made this class for my page and I thought I could share it.
This is a pretty simple and easy to use, template/page class.
What it simply does is; it adds content after a tag in the template file.
Aff, I suck at explaining. Download the example instead ^^.
Example:
example.rar
Class:
Feel free to give me rep++ ;D
OTLand rep:
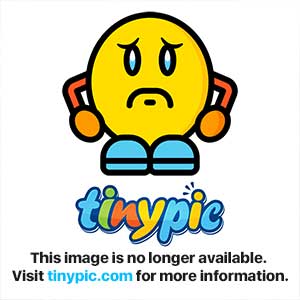
Simple Reputation System rep:
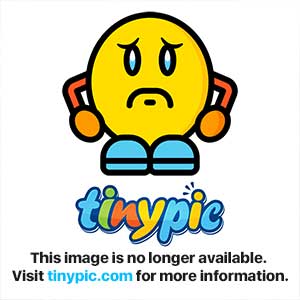
This is a pretty simple and easy to use, template/page class.
What it simply does is; it adds content after a tag in the template file.
Aff, I suck at explaining. Download the example instead ^^.
Example:
example.rar
Class:
PHP:
<?php
/*====================================================*\
|| ***************************************************||
|| * This file is part of Z-CMD 0.0.1
|| * -------------------------------------------------||
|| * Author website: http://zisly.com ||
|| * Extra credits:
|| Absolute Mango @otland for the preg_replace help
|| ***************************************************||
\*====================================================*/
defined( 'A_PAGE' ) or die( 'Restricted access' );
class page
{
// Holds the page content
private $page;
/* ===========================================================================================*\
|| __construct( )
|| $dir = path to theme
\* ===========================================================================================*/
public function __construct($dir)
{
$this->page = file_get_contents($dir.'index.html');
// Fixes the dirs inside the template file
$this->page = str_ireplace("{themedir?}", $dir, $this->page);
}
/* ===========================================================================================*\
|| parse ( )
|| $file = file to parse
\* ===========================================================================================*/
public function parse($file)
{
ob_start();
include($file);
$buffer = ob_get_contents();
ob_end_clean();
return $buffer;
}
/* ===========================================================================================*\
|| setdata ()
|| $tags = array of tags and their replacement
|| $bUnique = if the tag is unique
\* ===========================================================================================*/
public function setdata($tags, $bUnique = false)
{
// See if it's an array
if (is_array($tags))
{
// Start loop to start replacing tags
foreach ($tags as $tag => $data)
{
// Check if it's a files content the user wants to include
$data = (file_exists($data)) ? $this->parse($data) : $data;
// Checks if it's a unique element
$this->page = ($bUnique) ? preg_replace('/\{'.$tag.'\}/i',$data,$this->page): preg_replace('/\{'.$tag.'\}/i',$data.'{'.$tag.'}',$this->page);
}
}
}
/* =============================================================================================*\
|| output ()
|| Returns the page with all unused tags stripped
\* =============================================================================================*/
public function output()
{
// Clear all unused tags
$page = preg_replace('/\{(.*?)\}/i','',$this->page);
unset( $this );
// Returns page
return $page;
}
}
?>
OTLand rep:
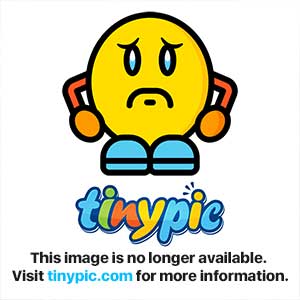
Simple Reputation System rep:
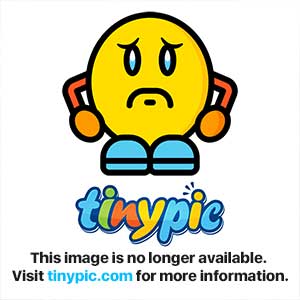
Last edited: