please somebody can help-me upgrade this system for tfs 1.4
ITEM_ATTRIBUTE_SPECIAL
otland.net
enums
events.cpp
item.cpp
luascript.cpp
movements.cpp
player.cpp
player.h
tools.h
C++:
enum SpecialSkills_t {
SPECIALSKILL_MAGICLEVEL = 1,
SPECIALSKILL_FIRST = SPECIALSKILL_MAGICLEVEL,
SPECIALSKILL_LAST = SPECIALSKILL_MAGICLEVEL
};
enum itemCustomAttrTypes : uint32_t {
ITEM_CUSTOMATTRIBUTE_SPECIALSKILL_MAGICLEVEL = 1,
};
events.cpp
C++:
bool Events::eventPlayerOnEquipItem(Player* player, Item* item, slots_t slot, bool isCheck)
{
// Player:onEquipItem(item, slot, isCheck)
if (info.playerOnEquipItem == -1) {
return true;
}
if (!scriptInterface.reserveScriptEnv()) {
std::cout << "[Error - Events::eventPlayerOnEquipItem] Call stack overflow" << std::endl;
return false;
}
ScriptEnvironment* env = scriptInterface.getScriptEnv();
env->setScriptId(info.playerOnEquipItem, &scriptInterface);
lua_State* L = scriptInterface.getLuaState();
scriptInterface.pushFunction(info.playerOnEquipItem);
LuaScriptInterface::pushUserdata<Player>(L, player);
LuaScriptInterface::setMetatable(L, -1, "Player");
LuaScriptInterface::pushUserdata<Item>(L, item);
LuaScriptInterface::setMetatable(L, -1, "Item");
lua_pushnumber(L, (int8_t)slot);
lua_pushboolean(L, isCheck);
return scriptInterface.callFunction(4);
}
bool Events::eventPlayerOnDeEquipItem(Player* player, Item* item, slots_t slot, bool isCheck)
{
// Player:onDeEquipItem(item, slot, isCheck)
if (info.playerOnDeEquipItem == -1) {
return true;
}
if (!scriptInterface.reserveScriptEnv()) {
std::cout << "[Error - Events::eventPlayerOnDeEquipItem] Call stack overflow" << std::endl;
return false;
}
ScriptEnvironment* env = scriptInterface.getScriptEnv();
env->setScriptId(info.playerOnDeEquipItem, &scriptInterface);
lua_State* L = scriptInterface.getLuaState();
scriptInterface.pushFunction(info.playerOnDeEquipItem);
LuaScriptInterface::pushUserdata<Player>(L, player);
LuaScriptInterface::setMetatable(L, -1, "Player");
LuaScriptInterface::pushUserdata<Item>(L, item);
LuaScriptInterface::setMetatable(L, -1, "Item");
lua_pushnumber(L, (int8_t)slot);
lua_pushboolean(L, isCheck);
return scriptInterface.callFunction(4);
}
bool eventPlayerOnEquipItem(Player* player, Item* item, slots_t slot, bool isCheck);
bool eventPlayerOnDeEquipItem(Player* player, Item* item, slots_t slot, bool isCheck);
C++:
void Item::setCustomAttribute(std::string& key, ItemAttributes::CustomAttribute& value)
{
int64_t tmpInt = atoi(key.c_str());
getAttributes()->setCustomAttribute(key, value);
if (tmpInt > 0) {
SpecialSkills_t skill = getCustomAttributeSpecialSkill((itemCustomAttrTypes)tmpInt);
if (skill > 0) {
Thing* thing = getTopParent() ? (Thing*)getTopParent() : nullptr;
if (thing)
if (Creature* creature = thing->getCreature()) {
if (Player* player = creature->getPlayer())
player->updateSpecialSkills();
}
}
}
}
(tmpStrValue == "specialskillmagiclevel") {
abilities.specialSkills[SPECIALSKILL_MAGICLEVEL] = pugi::cast<int32_t>(valueAttribute.value());
luascript.cpp
C++:
int LuaScriptInterface::luaPlayerGetSpecialSkill(lua_State* L)
{
// player:getSpecialSkill(specialSkillType)
SpecialSkills_t specialSkillType = getNumber<SpecialSkills_t>(L, 2);
Player* player = getUserdata<Player>(L, 1);
if (player && specialSkillType <= SPECIALSKILL_LAST) {
lua_pushnumber(L, player->getSpecialSkill(specialSkillType));
}
else {
lua_pushnil(L);
}
return 1;
}
int LuaScriptInterface::luaPlayerAddSpecialSkill(lua_State* L)
{
// player:addSpecialSkill(specialSkillType, value)
Player* player = getUserdata<Player>(L, 1);
if (!player) {
lua_pushnil(L);
return 1;
}
SpecialSkills_t specialSkillType = getNumber<SpecialSkills_t>(L, 2);
if (specialSkillType > SPECIALSKILL_LAST) {
lua_pushnil(L);
return 1;
}
player->addVarSpecialSkill(specialSkillType, getNumber<int32_t>(L, 3));
player->sendSkills();
pushBoolean(L, true);
return 1;
}
int LuaScriptInterface::luaItemTypeGetSpecialSkillMagicLevel(lua_State* L) {
// itemType:getSpecialSkillMagicLevel()
const ItemType* itemType = getUserdata<const ItemType>(L, 1);
if (itemType) {
lua_pushnumber(L, itemType->getSpecialSkillMagicLevel());
} else {
lua_pushnil(L);
}
return 1;
}
C++:
uint32_t MoveEvents::onPlayerEquip(Player* player, Item* item, slots_t slot, bool isCheck)
{
player->updateSpecialSkills();
if (g_events->eventPlayerOnEquipItem(player, item, slot, isCheck)) {
MoveEvent* moveEvent = getEvent(item, MOVE_EVENT_EQUIP, slot);
if (!moveEvent) {
return 1;
}
return moveEvent->fireEquip(player, item, slot, isCheck);
}
return false;
}
uint32_t MoveEvents::onPlayerDeEquip(Player* player, Item* item, slots_t slot)
{
player->updateSpecialSkills();
if (g_events->eventPlayerOnDeEquipItem(player, item, slot, false)) {
MoveEvent* moveEvent = getEvent(item, MOVE_EVENT_DEEQUIP, slot);
if (!moveEvent) {
return 1;
}
return moveEvent->fireEquip(player, item, slot, false);
}
return false;
}
C++:
void Player::updateSpecialSkills()
{
for (int32_t i = SPECIALSKILL_FIRST; i <= SPECIALSKILL_LAST; ++i) {
setVarSpecialSkill(static_cast<SpecialSkills_t>(i), 0);
}
for (uint8_t slot = CONST_SLOT_FIRST; slot < CONST_SLOT_LAST; slot++) {
Thing* thing = getThing(slot);
if (thing) {
Item* item = thing->getItem();
if (item) {
const ItemType& iType = Item::items[item->getID()];
for (int32_t i = SPECIALSKILL_FIRST; i <= SPECIALSKILL_LAST; ++i) {
if (iType.abilities->specialSkills[i]) {
int64_t skillValue = iType.abilities->specialSkills[i];
addVarSpecialSkill(static_cast<SpecialSkills_t>(i), skillValue);
}
const ItemAttributes::CustomAttribute* attr = item->getCustomAttribute(getSpecialSkillCustomAttribute(i));
if (attr) {
int64_t skillValue = boost::get<int64_t>(attr->value);
addVarSpecialSkill(static_cast<SpecialSkills_t>(i), skillValue);
}
}
}
}
}
sendSkills();
sendStats();
}
player.h
C++:
void addVarSpecialSkill(SpecialSkills_t skill, int32_t modifier) {
varSpecialSkills[skill] += modifier;
}
void setVarSpecialSkill(SpecialSkills_t skill, int32_t modifier)
{
varSpecialSkills[skill] = modifier;
}
void updateSpecialSkills();
uint16_t getSpecialSkill(uint8_t skill) const {
return std::max<int32_t>(0, varSpecialSkills[skill]);
}
C++:
itemCustomAttrTypes getSpecialSkillCustomAttribute(uint8_t skillid);
SpecialSkills_t getCustomAttributeSpecialSkill(itemCustomAttrTypes attr);
ITEM_ATTRIBUTE_SPECIAL
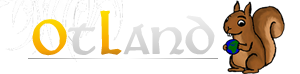
[LIB] Storing Information (Items and Players) TFS 1.2
The purpose of this lib is to add flexibility to what you're going to save in your server about players and items. For example, using this lib you can save any string, table, boolean or number in a player and/or in an item and it will be saved in the database. For the item part I had to edit...
Last edited: