Hello, I have followed this
OTCv8 detection, custom features packet, bigget packet size and bug f… · OTCv8/forgottenserver@2839d4d (https://github.com/OTCv8/forgottenserver/commit/2839d4d7a8ad3597eff6c786f4ceb9b1b4b4456b)'
Added early wings and aura support · OTCv8/forgottenserver@866a98f (https://github.com/OTCv8/forgottenserver/commit/866a98f13d17e36c33c3db107a65fc54c26203bf)
Early shaders supports, better aura & wings support · OTCv8/forgottenserver@2f494dc (https://github.com/OTCv8/forgottenserver/commit/2f494dcbe742a09afd6a3e93e5abcea8c821f8fd)
Crash bug fix for outfit shaders · OTCv8/forgottenserver@239f555 (https://github.com/OTCv8/forgottenserver/commit/239f555b0f71cfe0e17b6e5012518b25696d2f76)
In this order.
But still, Its 0 in aura and wings etc,
I added one aura and tried to buy it in shop, and i get this code
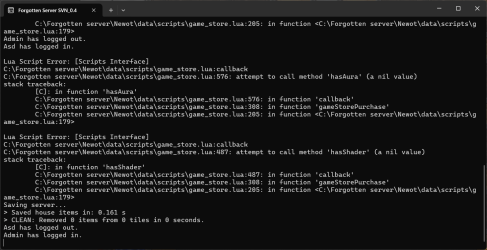
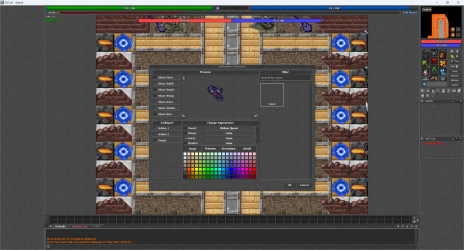
OTCv8 detection, custom features packet, bigget packet size and bug f… · OTCv8/forgottenserver@2839d4d (https://github.com/OTCv8/forgottenserver/commit/2839d4d7a8ad3597eff6c786f4ceb9b1b4b4456b)'
Added early wings and aura support · OTCv8/forgottenserver@866a98f (https://github.com/OTCv8/forgottenserver/commit/866a98f13d17e36c33c3db107a65fc54c26203bf)
Early shaders supports, better aura & wings support · OTCv8/forgottenserver@2f494dc (https://github.com/OTCv8/forgottenserver/commit/2f494dcbe742a09afd6a3e93e5abcea8c821f8fd)
Crash bug fix for outfit shaders · OTCv8/forgottenserver@239f555 (https://github.com/OTCv8/forgottenserver/commit/239f555b0f71cfe0e17b6e5012518b25696d2f76)
In this order.
But still, Its 0 in aura and wings etc,
I added one aura and tried to buy it in shop, and i get this code
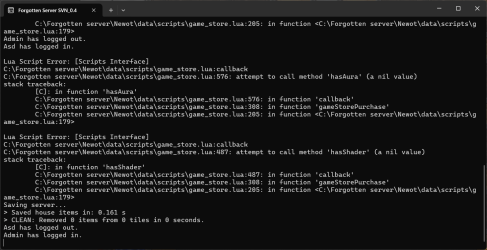
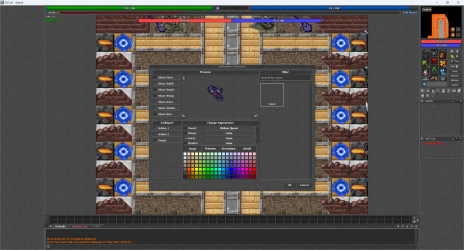