Is this how DeEquip is supposed to work with stackable items on TFS 1.4.2?
Shouldn't it only remove attributes when the whole stack is unEquipped?
Example: Using a normal spear for testing.
This is how it's added to items.xml:
This is how it's added to movements.xml:
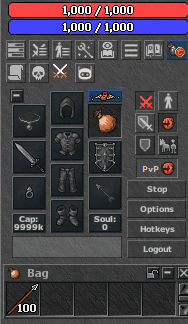
I've tried to add some checks on Lua on a DeEquip script but nothing worked.
How can I make it to only remove attributes when the whole item stack is unEquipped?
Shouldn't it only remove attributes when the whole stack is unEquipped?
Example: Using a normal spear for testing.
This is how it's added to items.xml:
XML:
<item id="2389" article="a" name="spear">
<attribute key="weight" value="2000" />
<attribute key="attack" value="25" />
<attribute key="weaponType" value="distance" />
<attribute key="shootType" value="spear" />
<attribute key="maxhitpoints" value="500" />
<attribute key="maxmanapoints" value="500" />
<attribute key="maxHitChance" value="76" />
<attribute key="range" value="3" />
<attribute key="showattributes" value="1" />
</item>
XML:
<movevent event="Equip" itemid="2389" slot="hand" function="onEquipItem" />
<movevent event="DeEquip" itemid="2389" slot="hand" function="onDeEquipItem" />
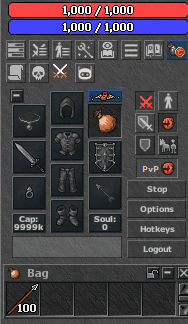
I've tried to add some checks on Lua on a DeEquip script but nothing worked.
How can I make it to only remove attributes when the whole item stack is unEquipped?
Last edited: