Two spells, for your enjoyment 
For both of the spells you'll need these two functions:
Put them in your global.lua, or in the spells themselves (or whereever).
Fire Nova
Fire nova, end of story.
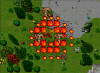
Lua
XML
Implosion
More or less the opposite of the previous spell.
LUA
XML
Playing around with the size variable obviously changes the radius of the circles. The damageformula variable is probably horrendous so you might want to play around with that as well.
Enjoy
Edit: Fire Nova is self-evidently a better name for the first spell.
For both of the spells you'll need these two functions:
Code:
function distanceTo(fromPos, toPos)
dx = toPos.x - fromPos.x
dy = toPos.y - fromPos.y
dz = toPos.z - fromPos.z
return math.sqrt(dx*dx + dy*dy + dz*dz)
end
function distanceToSquared(fromPos, toPos)
dx = toPos.x - fromPos.x
dy = toPos.y - fromPos.y
dz = toPos.z - fromPos.z
return dx*dx + dy*dy + dz*dz
end
Put them in your global.lua, or in the spells themselves (or whereever).
Fire Nova
Fire nova, end of story.
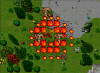
Lua
Code:
local size = 9
local center = {x = math.ceil(size/2), y = math.ceil(size/2), z = 0}
local effectType = CONST_ME_HITBYFIRE
local damageType = COMBAT_FIREDAMAGE
local combat = createCombatObject()
arr = {}
for i = 1,size do
arr[i] = {}
for j = 1,size do
if(distanceToSquared(center, {x = i, y = j, z = 0}) < (size*size/4)) then
arr[i][j] = 1
else
arr[i][j] = 0
end
end
end
arr[math.ceil(size/2)][math.ceil(size/2)] = 2;
local area = createCombatArea(arr)
setCombatArea(combat, area)
function fireNovaCallback(cid, pos)
local distance = distanceTo(getPlayerPosition(cid), pos)
local damageformula = -1e2*(1+0.1*getPlayerMagLevel(cid))/(distance)
addEvent(
function()
doSendMagicEffect(pos, effectType)
end, 200*distance, nil)
addEvent(
function()
doAreaCombatHealth(cid, damageType, pos, 0, damageformula, damageformula, effectType)
end, 200*distance, nil)
end
setCombatCallback(combat, CALLBACK_PARAM_TARGETTILE, "fireNovaCallback")
function onCastSpell(cid, var)
return doCombat(cid, combat, var)
end
XML
Code:
<instant name="Fire Nova" words="exevo mas orbis" lvl="50" manapercent="4" cooldown="1000" needlearn="0" script="nameofyourfile.lua">
<vocation name="Sorcerer"/>
</instant>
Implosion
More or less the opposite of the previous spell.
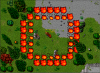
LUA
Code:
local size = 9
local center = {x = math.ceil(size/2), y = math.ceil(size/2), z = 0}
local effectType = CONST_ME_HITBYFIRE
local damageType = COMBAT_FIREDAMAGE
local combat = createCombatObject()
arr = {}
for i = 1,size do
arr[i] = {}
for j = 1,size do
if(distanceToSquared(center, {x = i, y = j, z = 0}) < (size*size/4)) then
arr[i][j] = 1
else
arr[i][j] = 0
end
end
end
arr[math.ceil(size/2)][math.ceil(size/2)] = 2;
local area = createCombatArea(arr)
setCombatArea(combat, area)
function implosionCallback(cid, pos)
local distance = distanceTo(getPlayerPosition(cid), pos)
local damageformula = -1e2*(1+0.1*getPlayerMagLevel(cid))/(distance)
if(distance < 2.5 and distance ~= 0) then
addEvent(
function()
doSendMagicEffect(pos, CONST_ME_CRAPS)
end, 100*(0.5 + size + distance), nil)
addEvent(
function()
doAreaCombatHealth(cid, COMBAT_EARTHDAMAGE, pos, 0, 2*damageformula, 2*damageformula, CONST_ME_HITAREA)
end, 100*(0.5 + size + distance), nil)
end
addEvent(
function()
doSendMagicEffect(pos, effectType)
end, 100*(0.5 + size - 2*distance), nil)
addEvent(
function()
doAreaCombatHealth(cid, damageType, pos, 0, damageformula, damageformula, effectType)
end, 100*(0.5 + size - 2*distance), nil)
end
setCombatCallback(combat, CALLBACK_PARAM_TARGETTILE, "implosionCallback")
function onCastSpell(cid, var)
return doCombat(cid, combat, var)
end
Code:
<instant name="Implosion" words="exevo implos" lvl="50" manapercent="4" cooldown="1000" needlearn="0" script="nameofyourspell.lua">
<vocation name="Sorcerer"/>
</instant>
Playing around with the size variable obviously changes the radius of the circles. The damageformula variable is probably horrendous so you might want to play around with that as well.
Enjoy
Edit: Fire Nova is self-evidently a better name for the first spell.
Last edited: