johnsamir
Advanced OT User
As title says. can someone tell me how do i convert this script from 0.4 to 1.5?
this is the normal script
know that cid isn't used anymore altough tfs 1.5 can handle it, changed cid to player but it hasn't worked either
also i saw this but it's not much explainatory
otland.net
thank
this is the normal script
LUA:
local storage = 54321
function onKill(cid, target)
local frags = getPlayerStorageValue(cid, storage)
if(isPlayer(target)) then
setPlayerStorageValue(cid, storage, frags+1)
if(frags == 1) then
doCreatureSetSkullType(cid, SKULL_YELLOW)
elseif(frags >= 2 and frags <= 4) then
doCreatureSetSkullType(cid, SKULL_GREEN)
elseif(frags >= 5 and frags <= 6) then
doCreatureSetSkullType(cid, SKULL_WHITE)
elseif(frags => 7) then
doCreatureSetSkullType(cid, SKULL_RED)
end
end
return true
end
also i saw this but it's not much explainatory
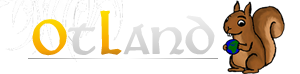
RevScripts - Tutorial to convert scripts from tfs 0.4 to 1.3
Is there any tutorial or tip to convert scripts from tfs 0.4 to 1.3?
thank