Mjmackan
Mapper ~ Writer
How to check players in X area for Y storage and then print the player with the highest amount of that storage in lua?
I need the player id, since i want to print the name of the player and the amount he has in Y storage.
TFS 1.3
I tried to use something like this..
And found this link..
stackoverflow.com
I didn't succeed that great, at best i printed nil hehe.
I need the player id, since i want to print the name of the player and the amount he has in Y storage.
TFS 1.3
I tried to use something like this..
LUA:
local fightAreas = Game.getSpectators({x = 930, y = 603, z = 7}, 10, 10, true)
if fightAreas ~= nil then
for _, areaPlayers in pairs(fightAreas) do
if isPlayer(areaPlayers) then
print(areaPlayers:getStorageValue(fragEventPlayer))
end
end
end
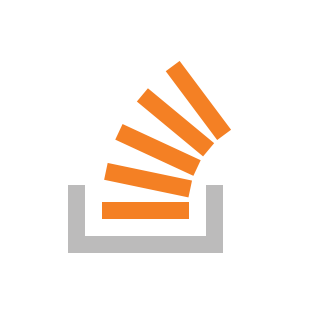
How do I get the highest integer in a table in Lua?
How do I get the highest integer in a table in Lua?
I didn't succeed that great, at best i printed nil hehe.
Last edited: