borisbrejcha
New Member
- Joined
- Jul 20, 2018
- Messages
- 9
- Reaction score
- 1
Good night, I'm trying to create a Pokeball class that extends the Item class ... However, I am having an error when I try to overwrite the Attr
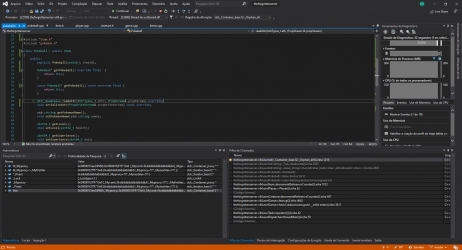
This error happens when I log out with my character, or try to delete the item with / r
This is my class Pokemon.h
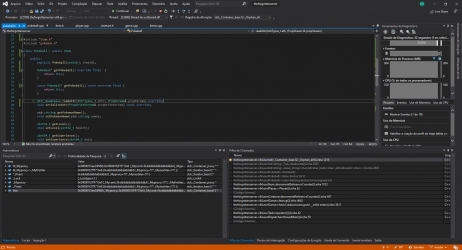
This error happens when I log out with my character, or try to delete the item with / r
This is my class Pokemon.h
C++:
/**
* The Forgotten Server - a free and open-source MMORPG server emulator
* Copyright (C) 2019 Mark Samman <[email protected]>
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along
* with this program; if not, write to the Free Software Foundation, Inc.,
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
*/
#ifndef FS_POKEBALL_H_009A319FB13D477D9EEFFBBD9BB83562
#define FS_POKEBALL_H_009A319FB13D477D9EEFFBBD9BB83562
#include "item.h"
#include "pokemon.h"
class Pokeball : public Item
{
public:
explicit Pokeball(uint16_t itemId);
Pokeball* getPokeball() override final {
return this;
}
const Pokeball* getPokeball() const override final {
return this;
}
Attr_ReadValue readAttr(AttrTypes_t attr, PropStream& propStream) override;
void serializeAttr(PropWriteStream& propWriteStream) const override;
std::string getPokemonName();
void setPokemonName(std::string name);
uint32_t getLevel();
void setLevel(uint32_t health);
uint64_t getExperience();
void setExperience(uint64_t exp);
int32_t getHealth();
void setHealth(int32_t health);
int32_t getMaxHealth();
void setMaxHealth(int32_t maxHealth);
Pokemon* getPokemon();
void setPokemon(Pokemon* monster);
private:
Pokemon* pokemon = nullptr;
std::string name = "";
std::string nick = "";
uint32_t level = 1;
uint64_t experience = 100;
int32_t health = 100;
int32_t healthMax = 100;
};
#endif
And Pokemon.cpp
Code:
/**
* The Forgotten Server - a free and open-source MMORPG server emulator
* Copyright (C) 2019 Mark Samman <[email protected]>
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along
* with this program; if not, write to the Free Software Foundation, Inc.,
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
*/
#include "otpch.h"
#include "pokeball.h"
#include "pokemon.h"
Pokeball::Pokeball(uint16_t itemId) : Item(itemId)
{
}
Attr_ReadValue Pokeball::readAttr(AttrTypes_t attr, PropStream& propStream)
{
switch (attr) {
case ATTR_POKEBALLINFO: {
if (!propStream.read<std::string>(name)) {
return ATTR_READ_ERROR;
}
return ATTR_READ_CONTINUE;
}
default:
break;
}
return Item::readAttr(attr, propStream);
}
void Pokeball::serializeAttr(PropWriteStream& propWriteStream) const
{
Item::serializeAttr(propWriteStream);
propWriteStream.write<uint8_t>(ATTR_POKEBALLINFO);
propWriteStream.write<std::string>(name);
}
std::string Pokeball::getPokemonName()
{
return name;
}
void Pokeball::setPokemonName(std::string nName)
{
name = nName;
}
uint32_t Pokeball::getLevel()
{
return level;
}
void Pokeball::setLevel(uint32_t lvl)
{
level = lvl;
}
uint64_t Pokeball::getExperience()
{
return experience;
}
void Pokeball::setExperience(uint64_t exp)
{
experience = exp;
}
int32_t Pokeball::getHealth()
{
return health;
}
void Pokeball::setHealth(int32_t nhealth)
{
health = nhealth;
}
int32_t Pokeball::getMaxHealth()
{
return healthMax;
}
void Pokeball::setMaxHealth(int32_t mhealth)
{
healthMax = mhealth;
}
Pokemon* Pokeball::getPokemon()
{
return pokemon;
}
void Pokeball::setPokemon(Pokemon* pk)
{
pokemon = pk;
}
In items.cpp I added this code snippet
C++:
if (it.id != 0) {
if (it.isDepot()) {
newItem = new DepotLocker(type);
} else if (it.isContainer()) {
newItem = new Container(type);
} else if (it.isTeleport()) {
newItem = new Teleport(type);
} else if (it.isMagicField()) {
newItem = new MagicField(type);
} else if (it.isDoor()) {
newItem = new Door(type);
} else if (it.isTrashHolder()) {
newItem = new TrashHolder(type);
} else if (it.isMailbox()) {
newItem = new Mailbox(type);
} else if (it.isBed()) {
newItem = new BedItem(type);
} else if (it.isPokeball()) {
newItem = new Pokeball(type);
} else if (it.id >= 2210 && it.id <= 2212) { // magic rings
newItem = new Item(type - 3, count);
} else if (it.id == 2215 || it.id == 2216) { // magic rings
newItem = new Item(type - 2, count);
} else if (it.id >= 2202 && it.id <= 2206) { // magic rings