I'm gonna get straight to the point and make this very simple. I had a thread already written up before this one, but I got too complex, if you want to get into the more complex stuff, feel free to post here, we can talk about it. Making this tutorial complex to understand is not my goal.
So, what is addEvent()?
It is a method that calls a function or evaluates an expression after a specified number of milliseconds.
In layman's terms, it runs code after X milliseconds.
Quick reminders:
All you need to remember is that addEvent() simply sets a wait time to the callback function before it executes.
So, whatever the function is in callback parameter, it will execute that after whatever the wait time is in the milliseconds parameter.
If the function in the callback parameter has any parameters itself, it needs to be included as additional parameters (...) in addEvent().
To put in pictures;
Learning by Example
Can you guess what this script does?
Remember, callback is the function that will be executed at the end of the wait time.
Additional parameters (...) are only required if the callback function requires parameters.
Another example with a walk-through...
Okay, so based on what you know so far, how can you accomplish this one?
Take a note on what is going on:
Next, we want to make the lever go to the right and disappear the pillar (with poff magic effect).
If we go back to the notes, a left lever (1945) and a right lever (1946) do two different things,
so we want to make sure we check for the lever before doing their respective jobs.
You should now have something like this
What's the last and most important thing we need left? The pillar to come back 3 seconds later.
When I pull the lever, the pillar disappears. From the moment it disappears, I want to start the 3 second wait time right away, so it must be within the block where it disappears the pillar.
Now we need to make the addEvent() do what we want it to do.
If you look at the notes under #3, we said the wall comes back (with magic effect) and lever is moved to the left
In this case, instead of making a separate function for the server to "save", we can just use lambda/anonymous functions in the callback parameter. Don't worry, it's super easy; in my second post, you can read more about it.
Constructing the addEvent() (step-by-step)
Focusing on the addEvent(), we should have this:
Inside this function(), we want the pillar to reappear (magic effect POFF too) and the lever to move back to the left.
In order to tell the function which position to create the pillar and send the POFF magic effect, we have to pass it into the function through a parameter.
We also need to 'get' the lever object in order to flip it back to the left.
Again, in order to tell the function which lever to 'get', we need to pass it as a parameter.
Now function() has all the information it needs, let's make it do what it needs to do!
TADA! With all this code
We have this!
PLEASE! If you have any questions, comments, concerns, please post here!
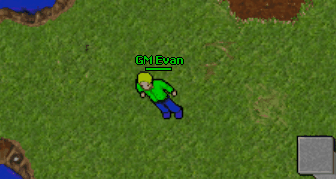
So, what is addEvent()?
It is a method that calls a function or evaluates an expression after a specified number of milliseconds.
In layman's terms, it runs code after X milliseconds.
Quick reminders:
- 1000 milliseconds = 1 second
- It is only executed once
- stopEvent() will prevent the function to run
- addEvent(callback, milliseconds, ...)
- callback - REQUIRED
- The function that will be executed
- milliseconds - REQUIRED
- The time in milliseconds to wait before executing the function
- ... - OPTIONAL
- Additional parameters to pass into the callback function
- callback - REQUIRED
- stopEvent(eventid)
- eventid - REQUIRED
- The eventid of the addEvent you're planning to end
- eventid - REQUIRED
All you need to remember is that addEvent() simply sets a wait time to the callback function before it executes.
So, whatever the function is in callback parameter, it will execute that after whatever the wait time is in the milliseconds parameter.
If the function in the callback parameter has any parameters itself, it needs to be included as additional parameters (...) in addEvent().
To put in pictures;
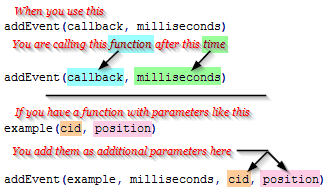
Learning by Example
LUA:
function sendHelloMessage(playerid)
local player = Player(playerid)
if not player then
return false
end
player:sendTextMessage(MESSAGE_STATUS_CONSOLE_BLUE, "Hello")
return true
end
function onUse(cid, item, fromPosition, itemEx, toPosition, isHotkey)
addEvent(sendHelloMessage, 3000, cid)
return true
end
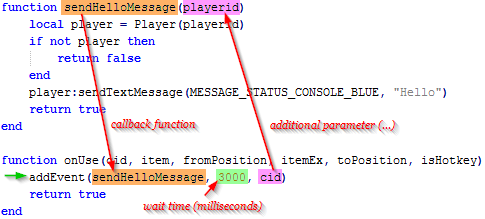
Can you guess what this script does?
It sends a blue-colored "Hello" message to the player's console, 3 seconds after using an object.
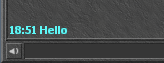
Remember, callback is the function that will be executed at the end of the wait time.
Additional parameters (...) are only required if the callback function requires parameters.
Another example with a walk-through...
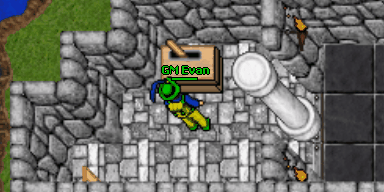
Okay, so based on what you know so far, how can you accomplish this one?
Take a note on what is going on:
- A pillar is blocking the path to the next room
- I pull lever to the right, pillar disappears (with magic effect)
- 3 seconds later, pillar comes back (with magic effect) and lever moves back to the left
- If the lever is to the right and you use it, nothing happens
LUA:
function onUse(cid, item, fromPosition, itemEx, toPosition, isHotkey)
return true
end
Next, we want to make the lever go to the right and disappear the pillar (with poff magic effect).
If we go back to the notes, a left lever (1945) and a right lever (1946) do two different things,
so we want to make sure we check for the lever before doing their respective jobs.
LUA:
function onUse(cid, item, fromPosition, itemEx, toPosition, isHotkey)
local pillarTile = Tile(X, Y, Z)
if item.itemid == 1945 then
pillarTile:getPosition():sendMagicEffect(CONST_ME_POFF)
pillarTile:getItemById(1515):remove()
Item(item.uid):transform(1946)
elseif item.itemid == 1946 then
return false
end
return true
end
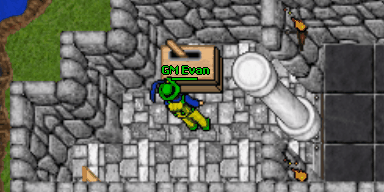
What's the last and most important thing we need left? The pillar to come back 3 seconds later.
When I pull the lever, the pillar disappears. From the moment it disappears, I want to start the 3 second wait time right away, so it must be within the block where it disappears the pillar.
LUA:
function onUse(cid, item, fromPosition, itemEx, toPosition, isHotkey)
local pillarTile = Tile(X, Y, Z)
if item.itemid == 1945 then
pillarTile:getPosition():sendMagicEffect(CONST_ME_POFF)
pillarTile:getItemById(1515):remove()
Item(item.uid):transform(1946)
addEvent(callback, milliseconds, ...) -- RIGHT HERE
elseif item.itemid == 1946 then
return false
end
return true
end
Now we need to make the addEvent() do what we want it to do.
If you look at the notes under #3, we said the wall comes back (with magic effect) and lever is moved to the left
In this case, instead of making a separate function for the server to "save", we can just use lambda/anonymous functions in the callback parameter. Don't worry, it's super easy; in my second post, you can read more about it.
Constructing the addEvent() (step-by-step)
Focusing on the addEvent(), we should have this:
LUA:
addEvent(function()
--
end, 3000)
Inside this function(), we want the pillar to reappear (magic effect POFF too) and the lever to move back to the left.
In order to tell the function which position to create the pillar and send the POFF magic effect, we have to pass it into the function through a parameter.
LUA:
addEvent(function(pillarPos)
--
end, 3000, pilarTile:getPosition())
We also need to 'get' the lever object in order to flip it back to the left.
Again, in order to tell the function which lever to 'get', we need to pass it as a parameter.
LUA:
addEvent(function(pillarPos, lever)
--
end, 3000, pillarTile:getPosition(), Tile(toPosition):getItemById(1946))
Now function() has all the information it needs, let's make it do what it needs to do!
LUA:
addEvent(function(pillarPos, lever)
Game.createItem(1515, 1, pillarPos) -- Create pillar
pillarPos:sendMagicEffect(CONST_ME_POFF) -- Send POFF effect
lever:transform(1945) -- Flip lever to the left
end, 3000, pillarTile:getPosition(), Tile(toPosition):getItemById(1946))
TADA! With all this code
LUA:
function onUse(cid, item, fromPosition, itemEx, toPosition, isHotkey)
local pillarTile = Tile(X, Y, Z)
if item.itemid == 1945 then
pillarTile:getPosition():sendMagicEffect(CONST_ME_POFF)
pillarTile:getItemById(1515):remove()
Item(item.uid):transform(1946)
addEvent(function(pillarPos, lever)
Game.createItem(1515, 1, pillarPos) -- Create pillar
pillarPos:sendMagicEffect(CONST_ME_POFF) -- Send POFF effect
lever:transform(1945) -- Flip lever to the left
end, 3000, pillarTile:getPosition(), Tile(toPosition):getItemById(1946))
elseif item.itemid == 1946 then
return false
end
return true
end
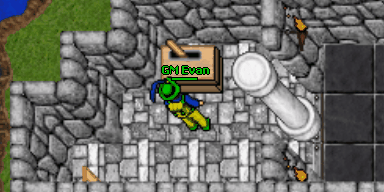
PLEASE! If you have any questions, comments, concerns, please post here!
Last edited by a moderator: