- Joined
- Nov 17, 2010
- Messages
- 6,798
- Solutions
- 581
- Reaction score
- 5,361
Create a custom shop via table.
Use that table to open a trade window.
In the example below I used simple keywords to show each different shop.. (Shop A, Shop B, Shop C (A & B merged))
But the uses of this is only limited by your imagination.
Few quick examples of things you could do
-> Different / merged shop based on input from player. (Think of the player saying potions / armor / runes, and then you filter the shop into those categories)
-> Gender based shop
-> Quest Status Shop (If quest requirements met, merge the shops / show different shop)
The onBuy and onSell functions are really simple and can be expanded upon, but that's not what I was worried about showcasing here.
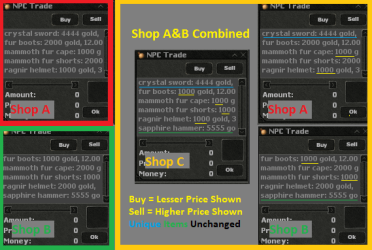
Use that table to open a trade window.
In the example below I used simple keywords to show each different shop.. (Shop A, Shop B, Shop C (A & B merged))
But the uses of this is only limited by your imagination.
Few quick examples of things you could do
-> Different / merged shop based on input from player. (Think of the player saying potions / armor / runes, and then you filter the shop into those categories)
-> Gender based shop
-> Quest Status Shop (If quest requirements met, merge the shops / show different shop)
The onBuy and onSell functions are really simple and can be expanded upon, but that's not what I was worried about showcasing here.
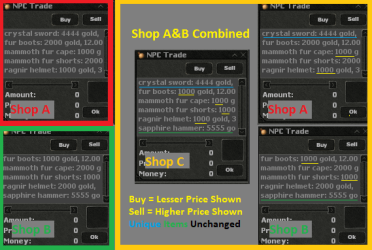
Lua:
local keywordHandler = KeywordHandler:new()
local npcHandler = NpcHandler:new(keywordHandler)
NpcSystem.parseParameters(npcHandler)
function onCreatureAppear(cid) npcHandler:onCreatureAppear(cid) end
function onCreatureDisappear(cid) npcHandler:onCreatureDisappear(cid) end
function onCreatureSay(cid, type, msg) npcHandler:onCreatureSay(cid, type, msg) end
function onThink() npcHandler:onThink() end
local shopA = {
{id=7462, buy=1000, sell=0, name='ragnir helmet'},
{id=7463, buy=1000, sell=0, name='mammoth fur cape'},
{id=7464, buy=2000, sell=0, name='mammoth fur shorts'},
{id=7457, buy=2000, sell=0, name='fur boots'},
{id=7449, buy=4444, sell=0, name='crystal sword'}
}
local shopB = {
{id=7462, buy=2000, sell=0, name='ragnir helmet'},
{id=7463, buy=2000, sell=0, name='mammoth fur cape'},
{id=7464, buy=1000, sell=0, name='mammoth fur shorts'},
{id=7457, buy=1000, sell=0, name='fur boots'},
{id=7437, buy=5555, sell=0, name='sapphire hammer'}
}
local function getTradeItems(t)
local list, obj = {}
for i = 1, #t do
obj = t[i]
list[obj.id] = {id = obj.id, buy = obj.buy, sell = obj.sell, name = ItemType(obj.id):getName():lower()}
end
return list
end
local function joinTables(old, new, mergeSameItems)
for k, v in pairs(new) do
if mergeSameItems then
local found = false
for _k, _v in pairs(old) do
if v.id == _v.id then
found = true
if v.buy < _v.buy then
_v.buy = v.buy
end
if v.sell > _v.sell then
_v.sell = v.sell
end
break
end
end
if not found then
old[#old + 1] = v
end
else
old[#old + 1] = v
end
end
return old
end
local function greetCallback(cid)
local player = Player(cid)
-- npcHandler:setMessage(MESSAGE_GREET, 'Welcome back old chap. What brings you here this time?')
return true
end
local function creatureSayCallback(cid, type, msg)
if not npcHandler:isFocused(cid) then return false end
local player = Player(cid)
if msgcontains("shopA", msg) then
local tradeItems = getTradeItems(shopA)
openShopWindow(cid, tradeItems, onBuy, onSell)
npcHandler:say("This is Shop A.", cid)
elseif msgcontains("shopB", msg) then
local tradeItems = getTradeItems(shopB)
openShopWindow(cid, tradeItems, onBuy, onSell)
npcHandler:say("This is Shop B.", cid)
elseif msgcontains("shopC", msg) then
local tradeItems = getTradeItems(shopA)
tradeItems = joinTables(tradeItems, shopB, true)
openShopWindow(cid, tradeItems, onBuy, onSell)
npcHandler:say("This is Shop C. (Shop A & Shop B combined.)", cid)
end
return true
end
local function onBuy(cid, item, subType, amount, ignoreCap, inBackpacks)
local player = Player(cid)
if not ignoreCap and player:getFreeCapacity() < ItemType(items[item].id):getWeight(amount) then
return player:sendTextMessage(MESSAGE_INFO_DESCR, 'You don\'t have enough capacity.')
end
if items[item].buy then
player:removeTotalMoney(amount * items[item].buy)
player:addItem(items[item].id, amount)
return player:sendTextMessage(MESSAGE_INFO_DESCR, 'Bought '..amount..'x '..items[item].name..' for '..items[item].buy * amount..' gold coins.')
end
return true
end
local function onSell(cid, item, subType, amount, ignoreCap, inBackpacks)
local player = Player(cid)
if items[item].sell then
player:addMoney(items[item].sell * amount)
player:removeItem(items[item].id, amount)
return player:sendTextMessage(MESSAGE_INFO_DESCR, 'Sold '..amount..'x '..items[item].name..' for '..items[item].sell * amount..' gold coins.')
end
return true
end
-- npcHandler:setMessage(MESSAGE_FAREWELL, 'Happy hunting, old chap!')
npcHandler:setCallback(CALLBACK_GREET, greetCallback)
npcHandler:setCallback(CALLBACK_MESSAGE_DEFAULT, creatureSayCallback)
npcHandler:addModule(FocusModule:new())