Meh I was asked to do that in the college so I did it. Using PPlay that is a framework(module based on pygame) developed at my college http://www2.ic.uff.br/pplay/ (The website is broken if you select english... the fuck)
I had to do it in 3 hours. Made in 2 so the code is a freakin garbage.
Code: (http://pastebin.com/4m9sCfrk)
Code + Resources: https://mega.nz/#!Tk4hFKgZ!JVJUovn9wY2YZlYXRAk7hS1rZ6JakdXjhrwexIcHRFo
I had to do it in 3 hours. Made in 2 so the code is a freakin garbage.
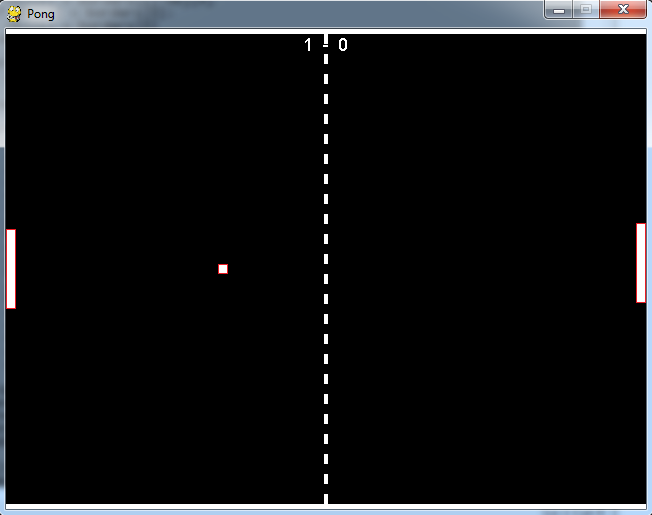
Code: (http://pastebin.com/4m9sCfrk)
Code + Resources: https://mega.nz/#!Tk4hFKgZ!JVJUovn9wY2YZlYXRAk7hS1rZ6JakdXjhrwexIcHRFo
Code:
from PPlay.window import *
from PPlay.sprite import *
from math import cos, sin, sqrt
Pi = 3.14159265359
font = pygame.font.Font("arial.ttf", 32)
scorefont = pygame.font.Font("arial.ttf", 18)
starttext = font.render("Press Space to Start!", 0,(255,255,255))
wintext = font.render("You win!", 0,(255,255,255))
losetext = font.render("You lose!", 0,(255,255,255))
scoreA = 0
scoreB = 0
class Game:
janela = False
screen = False
width = 0
heigth = 0
objetos = []
textobjs = []
keybinds = []
eventbinds = []
background = False
teclado = False
borders = False
def __init__(self, w, h):
self.janela = Window(w, h)
self.screen = self.janela.get_screen()
self.teclado = self.janela.get_keyboard()
self.borders = [0, w, 0, h]
self.width = w
self.heigth = h
def update(self):
if(self.janela):
if(self.background):
if(type(self.background) == tuple or type(self.background) == list):
self.screen.fill(self.background)
else:
self.background.draw()
for bind in self.eventbinds:
if(type(bind[1]) == int and bind[2]):
bind[0](self, self.objetos[bind[1]], bind[2])
elif(type(bind[1]) == int):
bind[0](self, self.objetos[bind[1]])
else:
bind[0](self)
for bind in self.keybinds:
if(bind[1]):
bind[0](self, self.teclado, self.objetos[bind[1]])
else:
bind[0](self, self.teclado)
for obj in self.objetos:
obj[0].set_position(obj[1], obj[2])
obj[0].draw()
for textobj in self.textobjs:
game.screen.blit(textobj[0], textobj[1])
self.janela.update()
def setbackground(self, obj):
self.background = obj
def setborders(self, borders):
self.borders = borders
def addobject(self, obj, w = 0, h = 0, posx = 0, posy = 0, v = [0, 0]):
#[obj, posx, posy, vetor, w, h, id]
obj.set_position(posx, posy)
tmp = []
tmp.append(obj)
tmp.append(posx)
tmp.append(posy)
tmp.append(v)
tmp.append(w)
tmp.append(h)
tmp.append(len(self.objetos))
self.objetos.append(tmp)
return len(self.objetos) - 1
def addtextobj(self, obj, pos):
self.textobjs.append([obj, pos])
def bindkeyboard(self, f, objid = False):
#self.janela, self.teclado, self.objetos[objid]
self.keybinds.append([f, objid])
return len(self.keybinds) - 1
def bindevent(self, f, objid = False, args = False):
self.eventbinds.append([f, objid, args])
# Inicio do Jogo:
def moverbat(game, teclado, obj):
janela = game.janela
borders = game.borders
if teclado.key_pressed("down"):
obj[2] += obj[3][1] * janela.delta_time()
if teclado.key_pressed("up"):
obj[2] -= obj[3][1] * janela.delta_time()
if obj[1] > borders[1]-obj[4]:
obj[1] = borders[1]-obj[4]
elif obj[1] < borders[0]:
obj[1] = borders[0]
if obj[2] > borders[3]-obj[5]:
obj[2] = borders[3]-obj[5]
elif obj[2] < borders[2]:
obj[2] = borders[2]
return
def moverbat2(game, teclado, obj):
janela = game.janela
borders = game.borders
if teclado.key_pressed("s"):
obj[2] += obj[3][1] * janela.delta_time()
if teclado.key_pressed("w"):
obj[2] -= obj[3][1] * janela.delta_time()
if obj[1] > borders[1]-obj[4]:
obj[1] = borders[1]-obj[4]
elif obj[1] < borders[0]:
obj[1] = borders[0]
if obj[2] > borders[3]-obj[5]:
obj[2] = borders[3]-obj[5]
elif obj[2] < borders[2]:
obj[2] = borders[2]
return
def speed(vx, vy):
return sqrt(vx**2 + vy**2)
def endGame(game, win):
text = wintext if win else losetext
game.objetos = []
game.keybinds = []
game.eventbinds = []
game.textobjs = []
game.setbackground([0, 0, 0])
game.addtextobj(text, [(game.width-text.get_width())/2, (game.heigth-text.get_height())/2])
game.bindkeyboard(startGame)
game.setbackground((0, 0, 0))
game.addtextobj(starttext, [(game.width-starttext.get_width())/2, (game.heigth-starttext.get_height())/2 + 40])
def moverball(game, obj):
janela = game.janela
borders = game.borders
for objc in game.objetos:
if objc[6] != obj[6]:
if(obj[7] != objc[6] and obj[0].collided(objc[0])):
f = obj[5]/2
a = 1
b = 1
if(obj[3][0] > 0):
a = -1
b = 1
f = 0
else:
a = 1
b = -1
intersect = (objc[2]+(objc[5]/2)) - (obj[2] + f)
nintersect = (intersect/(objc[5]/2))
obj[8] = nintersect * 5*Pi/12
curspeed = speed(obj[3][0], obj[3][1])
obj[3][0] = curspeed*cos(obj[8])*a
obj[3][1] = curspeed*sin(obj[8])*b
obj[7] = objc[6]
break
obj[1] += obj[3][0] * janela.delta_time()
obj[2] += obj[3][1] * janela.delta_time()
if obj[1] > borders[1]-obj[4]:
endGame(game, True)
global scoreA
scoreA = scoreA + 1
elif obj[1] < borders[0]:
endGame(game, False)
global scoreB
scoreB = scoreB + 1
if obj[2] > borders[3]-obj[5]:
obj[2] = borders[3]-obj[5]
obj[3][1] = -obj[3][1]
elif obj[2] < borders[2]:
obj[2] = borders[2]
obj[3][1] = -obj[3][1]
return
def moverbatia(game, obj, args):
ballobj = game.objetos[args[0]]
janela = game.janela
borders = game.borders
if ballobj[2] > obj[2] + obj[5]/2:
obj[2] += obj[3][1] * janela.delta_time()
if ballobj[2] < obj[2] + obj[5]/2:
obj[2] -= obj[3][1] * janela.delta_time()
if obj[1] > borders[1]-obj[4]:
obj[1] = borders[1]-obj[4]
elif obj[1] < borders[0]:
obj[1] = borders[0]
if obj[2] > borders[3]-obj[5]:
obj[2] = borders[3]-obj[5]
elif obj[2] < borders[2]:
obj[2] = borders[2]
return
def startGame(game, teclado):
if teclado.key_pressed("space"):
game.keybinds = []
game.textobjs = []
game.setborders([0, 640, 5, 475])
game.setbackground(Sprite("background.png"))
scoretext = scorefont.render(str(scoreA) + " - " + str(scoreB), 0,(255,255,255))
game.addtextobj(scoretext, [(game.width-scoretext.get_width())/2, 5])
ball = game.addobject(Sprite("ball.png"), 10, 10, 315, 235, [-400, 0])
game.objetos[ball].append(-1) # last collision
game.objetos[ball].append(0) # angle
batone = game.addobject(Sprite("bat.png"), 10, 80, 0, 200, [0, 300])
game.objetos[batone].append(0)
battwo = game.addobject(Sprite("bat.png"), 10, 80, 630, 200, [0, 310])
game.objetos[battwo].append(0)
game.bindkeyboard(moverbat, batone)
#game.bindevent(moverbatia, batone, [ball])
game.bindevent(moverbatia, battwo, [ball])
game.bindevent(moverball, ball)
game = Game(640, 480)
game.janela.set_title("Pong")
game.bindkeyboard(startGame)
game.setbackground((0, 0, 0))
game.addtextobj(starttext, [(game.width-starttext.get_width())/2, (game.heigth-starttext.get_height())/2])
while(1):
game.update()