I really don't have the time to rewrite this and its built in a separate framework than that of TFS but I figured its useful to whomever would like to make simple animation sequences for their special effects with spells or attacks...
I thought about rebuilding it so it used keyframes as entire area but currently it only uses one position per frame.
Please excuse the poorly written code
I wrote it when I was 1st learning lua and the love2d framework.
What does this do? It creates a table of tables which correspond to the entire width and height of the default game window.
Which then can be added to this script.
And then you can call it via spell (just an example)
You will need to download and install love2d for your platform (Mac, Windows or Linux) to run this tiny program. If you want it to run without using batch file then just rename the extension from zip to love.
I was using spell creator, the one found here on otland but idk i figured screw i should work on the program i wanted to make, this is a test of loading the sprite animations.
I am only loading 7 animation sequences and just to see if they lag at all, they automatically load from the effects directory.
They are the png files you get when you export a sprite.
Making progress, added a grid for clarity
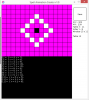
I thought about rebuilding it so it used keyframes as entire area but currently it only uses one position per frame.
Please excuse the poorly written code

What does this do? It creates a table of tables which correspond to the entire width and height of the default game window.
LUA:
animation = {
{1, 1}, -- index 1
{2, 2}, -- index 2
{3, 3}, -- index 3
{4, 3}, -- index 4
{5, 3}, -- index 5
{6, 3}, -- index 6
{7, 3}, -- index 7
{8, 3}, -- index 8
{10, 3}, -- index 9
{10, 5}, -- index 10
{10, 7}, -- index 11
{10, 9}, -- index 12
{10, 10}, -- index 13
{10, 12}, -- index 14
{10, 13}, -- index 15
{8, 13}, -- index 16
{7, 13}, -- index 17
}
LUA:
local grid =
{
-- 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 1
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 2
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 3
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 4
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 5
{0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0}, -- 6
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 7
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 8
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 9
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 10
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}, -- 11
}
function deepcopy(orig)
local orig_type = type(orig)
local copy
if orig_type == 'table' then
copy = {}
for orig_key, orig_value in next, orig, nil do
copy[deepcopy(orig_key)] = deepcopy(orig_value)
end
setmetatable(copy, deepcopy(getmetatable(orig)))
else -- number, string, boolean, etc
copy = orig
end
return copy
end
function setSequence(t, d)
local x = {}
for n = 1, #d do
x[n] = deepcopy(t)
end
for i = 1, #x do
x[i][d[i][1]][d[i][2]] = 1
end
return x
end
LUA:
local areaSequence = setSequence(grid, animation)
local combat = {}
for x = 1, #areaSequence do
combat[x] = Combat()
combat[x]:setParameter(COMBAT_PARAM_TYPE, COMBAT_NONE)
combat[x]:setParameter(COMBAT_PARAM_EFFECT, CONST_ME_HOLYDAMAGE)
combat[x]:setArea(createCombatArea(areaSequence[x]))
end
local int = 1000
local xcute, xtime = 10, 500
function onCastSpell(creature, variant, isHotkey)
for f = 1, xcute do
addEvent(function()
for n = 1, #areaSequence do
addEvent(function(cid, var, i)
local c = Creature(cid)
if c then
if combat[i] then
combat[i]:execute(c, var)
end
end
end, n * int, creature:getId(), variant, n
)
end
end, f * xtime)
end
return true
end
You will need to download and install love2d for your platform (Mac, Windows or Linux) to run this tiny program. If you want it to run without using batch file then just rename the extension from zip to love.
I was using spell creator, the one found here on otland but idk i figured screw i should work on the program i wanted to make, this is a test of loading the sprite animations.
I am only loading 7 animation sequences and just to see if they lag at all, they automatically load from the effects directory.
They are the png files you get when you export a sprite.
Making progress, added a grid for clarity
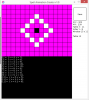
Attachments
-
Spell-Animation-Creator.zip5.4 KB · Views: 37 · VirusTotal
Last edited by a moderator: