- Joined
- Mar 16, 2017
- Messages
- 1,459
- Solutions
- 163
- Reaction score
- 2,152
- Location
- London
- GitHub
- MillhioreBT
- YouTube
- millhiorebt
Extra: Most updated version with new features
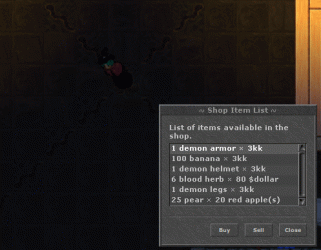
Note: Versión custom (Use the Extra)
A small store system with command but that also works with windows.
If you want to open the modalWindow you just have to type:
If you want to buy with just the command you must type:
The modalWindow will only show 255 items, I haven't added a page system yet, but I think 255 is more than enough.
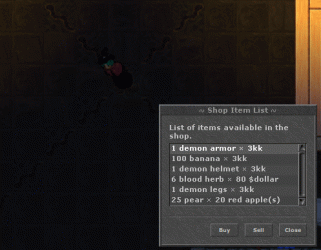
Note: Versión custom (Use the Extra)
A small store system with command but that also works with windows.
If you want to open the modalWindow you just have to type:
!shop list
If you want to buy with just the command you must type:
!shop <nombre del articulo>
The modalWindow will only show 255 items, I haven't added a page system yet, but I think 255 is more than enough.
LUA:
local shop = {}
local modalWindow = ModalWindow(99999, "~ Shop Item List ~", "List of items available in the shop.")
modalWindow:addButton(1, "Buy")
modalWindow:addButton(2, "Close")
modalWindow:setDefaultEscapeButton(2)
modalWindow:setDefaultEnterButton(2)
local index = 1
for name, item in pairs({
-- The names are converted to lowercase letters automatically, don't worry.
-- Note: for stackable items the maximum quantity is 100, and for non-stackable items the maximum quantity is 1
-- Examples:
["Demon Helmet"] = { id=2493, price=3000000 },
["Demon Armor"] = { id=2494, price=3000000 },
["Demon Legs"] = { id=2495, price=3000000 },
["Banana"] = { id=2676, price=3000000, count=100 } -- item stackable
}) do
local lowerCaseName = name:lower()
if index <= 255 then -- This is due to the client's limitations, in the future I may improve this system so that it has pages, but for now that is all
modalWindow:addChoice(index, string.format("[ %s x%d ]: %d gold coins.", lowerCaseName, math.min(item.count and item.count or 1, 100), item.price))
shop[index] = lowerCaseName -- for help to modalWindow
index = index +1
end
shop[lowerCaseName] = item
end
local function buyItem(player, param)
local item = shop[param:lower()]
if not item then
player:sendCancelMessage(string.format("Item witi name %s not found!", param))
return false
end
local money = player:getMoney()
local bankBalance = player:getBankBalance()
local totalMoney = money + bankBalance
if totalMoney < item.price then
player:sendCancelMessage(string.format("You don't have enough gold coins, You need %d gold coins.", item.price))
return false
end
local buyedItem = Game.createItem(item.id, math.min((item.count and item.count or 1), 100))
if not buyedItem or not buyedItem:getType():isMovable() then
print(string.format("Warning: The shop item with ID: %d is invalid.\n%s", item.id, debug.traceback()))
Item.remove(buyedItem) -- in case the buyedItem is nil, no problem will occur
return false
end
if player:addItemEx(buyedItem) ~= RETURNVALUE_NOERROR then
player:sendCancelMessage(RETURNVALUE_NOTENOUGHCAPACITY)
buyedItem:remove()
return false
end
local resultMoney = money - item.price
if resultMoney < 0 then
player:removeMoney(money)
player:setBankBalance(bankBalance + resultMoney)
else
player:removeMoney(item.price)
end
player:getPosition():sendMagicEffect(CONST_ME_FIREWORK_YELLOW)
player:sendTextMessage(MESSAGE_STATUS_CONSOLE_BLUE, string.format("Your purchase was successful! (%s x%d).\nYou now have %d gold coins and %d on the bank balance.", buyedItem:getName(), buyedItem:getCount(), money, player:getBankBalance()))
return false
end
local talkAction = TalkAction("!shop")
function talkAction.onSay(player, words, param, type)
if param == "list" then
modalWindow:sendToPlayer(player)
return false
end
return buyItem(player, param)
end
talkAction:separator(" ")
talkAction:register()
local creatureEvent = CreatureEvent("shopModalWindow")
function creatureEvent.onModalWindow(player, modalWindowId, buttonId, choiceId)
if modalWindowId == 99999 then
if buttonId == 1 then
local param = shop[choiceId]
if not param then
return true
end
buyItem(player, param)
return true
end
end
return true
end
creatureEvent:register()
local creatureEvent = CreatureEvent("shopRegister")
function creatureEvent.onLogin(player)
player:registerEvent("shopModalWindow")
return true
end
creatureEvent:register()
Last edited: