Daniel Kopeć
Member
The Status server does not show the number of players online.
How to fix it?
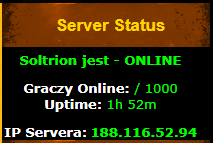
Gesior Acc Maker:
var/www/pot/OTS_ServerStatus.php
maybe there is something wrong here:
layout.php
How to fix it?
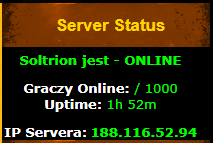
Gesior Acc Maker:
var/www/pot/OTS_ServerStatus.php
PHP:
<?php
/**#@+
* @version 0.1.4
* @since 0.1.4
*/
/**
* @package POT
* @author Wrzasq <[email protected]>
* @copyright 2007 (C) by Wrzasq
* @license http://www.gnu.org/licenses/lgpl-3.0.txt GNU Lesser General Public License, Version 3
*/
/**
* Wrapper for binary server status request.
*
* @package POT
* @property-read int $uptime Uptime.
* @property-read string $ip IP number.
* @property-read string $name Server name.
* @property-read int $port Server port.
* @property-read string $location Server physical location.
* @property-read string $url Website URL.
* @property-read string $serverVersion Server version.
* @property-read string $owner Owner name.
* @property-read string $eMail Owner's e-mail.
* @property-read int $onlinePlayers Players online count.
* @property-read int $maxPlayers Maximum allowed players count.
* @property-read int $playersPeak Record of players online.
* @property-read string $mapName Map name.
* @property-read string $mapAuthor Map author.
* @property-read int $mapWidth Map width.
* @property-read int $mapHeight Map height.
* @property-read string $motd Message Of The Day.
* @property-read array $players Online players list.
*/
class OTS_ServerStatus
{
/**
* Basic server info.
*/
const REQUEST_BASIC_SERVER_INFO = 1;
/**
* Server owner info.
*/
const REQUEST_OWNER_SERVER_INFO = 2;
/**
* Server extra info.
*/
const REQUEST_MISC_SERVER_INFO = 4;
/**
* Players stats info.
*/
const REQUEST_PLAYERS_INFO = 8;
/**
* Map info.
*/
const REQUEST_MAP_INFO = 16;
/**
* Extended players info.
*/
const REQUEST_EXT_PLAYERS_INFO = 32;
/**
* Player status info.
*/
const REQUEST_PLAYER_STATUS_INFO = 64;
/**
* Basic server respond.
*/
const RESPOND_BASIC_SERVER_INFO = 0x10;
/**
* Server owner respond.
*/
const RESPOND_OWNER_SERVER_INFO = 0x11;
/**
* Server extra respond.
*/
const RESPOND_MISC_SERVER_INFO = 0x12;
/**
* Players stats respond.
*/
const RESPOND_PLAYERS_INFO = 0x20;
/**
* Map respond.
*/
const RESPOND_MAP_INFO = 0x30;
/**
* Extended players info.
*/
const RESPOND_EXT_PLAYERS_INFO = 0x21;
/**
* Player status info.
*/
const RESPOND_PLAYER_STATUS_INFO = 0x22;
/**
* Server name.
*
* @var string
*/
private $name;
/**
* Server IP.
*
* @var string
*/
private $ip;
/**
* Server port.
*
* @var string
*/
private $port;
/**
* Owner name.
*
* @var string
*/
private $owner;
/**
* Owner's e-mail.
*
* @var string
*/
private $eMail;
/**
* Message of the day.
*
* @var stirng
*/
private $motd;
/**
* Server location.
*
* @var string
*/
private $location;
/**
* Website URL.
*
* @var stirng
*/
private $url;
/**
* Uptime.
*
* @var int
*/
private $uptime;
/**
* Status version.
*
* @var string
*/
private $version;
/**
* Players online.
*
* @var int
*/
private $online;
/**
* Maximum players.
*
* @var int
*/
private $max;
/**
* Players peak.
*
* @var int
*/
private $peak;
/**
* Map name.
*
* @var string
*/
private $map;
/**
* Map author.
*
* @var string
*/
private $author;
/**
* Map width.
*
* @var int
*/
private $width;
/**
* Map height.
*
* @var int
*/
private $height;
/**
* Players online list.
*
* @var array
*/
private $players = array();
/**
* Reads info from respond packet.
*
* @param OTS_Buffer $info Information packet.
*/
public function __construct(OTS_Buffer $info)
{
// skips packet length
$info->getShort();
while( $info->isValid() )
{
switch( $info->getChar() )
{
case self::RESPOND_BASIC_SERVER_INFO:
$this->name = $info->getString();
$this->ip = $info->getString();
$this->port = (int) $info->getString();
break;
case self::RESPOND_OWNER_SERVER_INFO:
$this->owner = $info->getString();
$this->eMail = $info->getString();
break;
case self::RESPOND_MISC_SERVER_INFO:
$this->motd = $info->getString();
$this->location = $info->getString();
$this->url = $info->getString();
$uptime = $info->getLong() << 32;
$this->uptime += $info->getLong() + $uptime;
$this->version = $info->getString();
break;
case self::RESPOND_PLAYERS_INFO:
$this->online = $info->getLong();
$this->max = $info->getLong();
$this->peak = $info->getLong();
break;
case self::RESPOND_MAP_INFO:
$this->map = $info->getString();
$this->author = $info->getString();
$this->width = $info->getShort();
$this->height = $info->getShort();
break;
case self::RESPOND_EXT_PLAYERS_INFO:
$count = $info->getLong();
for($i = 0; $i < $count; $i++)
{
$name = $info->getString();
$this->players[$name] = $info->getLong();
}
break;
}
}
}
/**
* Returns server uptime.
*
* @return int Uptime.
*/
public function getUptime()
{
return $this->uptime;
}
/**
* Returns server IP.
*
* @return string IP.
*/
public function getIP()
{
return $this->ip;
}
/**
* Returns server name.
*
* @return string Name.
*/
public function getName()
{
return $this->name;
}
/**
* Returns server port.
*
* @return int Port.
*/
public function getPort()
{
return $this->port;
}
/**
* Returns server location.
*
* @return string Location.
*/
public function getLocation()
{
return $this->location;
}
/**
* Returns server website.
*
* @return string Website URL.
*/
public function getURL()
{
return $this->url;
}
/**
* Returns server version.
*
* @return string Version.
*/
public function getServerVersion()
{
return $this->version;
}
/**
* Returns owner name.
*
* @return string Owner name.
*/
public function getOwner()
{
return $this->owner;
}
/**
* Returns owner e-mail.
*
* @return string Owner e-mail.
*/
public function getEMail()
{
return $this->eMail;
}
/**
* Returns current amount of players online.
*
* @return int Count of players.
*/
public function getOnlinePlayers()
{
return $this->online;
}
/**
* Returns maximum amount of players online.
*
* @return int Maximum allowed count of players.
*/
public function getMaxPlayers()
{
return $this->max;
}
/**
* Returns record of online players.
*
* @return int Players online record.
*/
public function getPlayersPeak()
{
return $this->peak;
}
/**
* Returns map name.
*
* @return string Map name.
*/
public function getMapName()
{
return $this->map;
}
/**
* Returns map author.
*
* @return string Mapper name.
*/
public function getMapAuthor()
{
return $this->author;
}
/**
* Returns map width.
*
* @return int Map width.
*/
public function getMapWidth()
{
return $this->width;
}
/**
* Returns map height.
*
* @return int Map height.
*/
public function getMapHeight()
{
return $this->height;
}
/**
* Returns server's Message Of The Day
*
* @return string Server MOTD.
*/
public function getMOTD()
{
return $this->motd;
}
/**
* Returns list of players currently online.
*
* @return array List of players in format 'name' => level.
*/
public function getPlayers()
{
}
/**
* Magic PHP5 method.
*
* @param string $name Property name.
* @return mixed Property value.
* @throws OutOfBoundsException For non-supported properties.
*/
public function __get($name)
{
switch($name)
{
case 'uptime':
return $this->getUptime();
case 'ip':
return $this->getIP();
case 'name':
return $this->getName();
case 'port':
return $this->getPort();
case 'location':
return $this->getLocation();
case 'url':
return $this->getURL();
case 'serverVersion':
return $this->getServerVersion();
case 'owner':
return $this->getOwner();
case 'eMail':
return $this->getEMail();
case 'onlinePlayers':
return $this->getOnlinePlayers();
case 'maxPlayers':
return $this->getMaxPlayers();
case 'playersPeak':
return $this->getPlayersPeak();
case 'mapName':
return $this->getMapName();
case 'mapAuthor':
return $this->getMapAuthor();
case 'mapWidth':
return $this->getMapWidth();
case 'mapHeight':
return $this->getMapHeight();
case 'motd':
return $this->getMOTD();
case 'players':
return $this->getPlayers();
default:
throw new OutOfBoundsException();
}
}
}
/**#@-*/
?>
Post automatically merged:
maybe there is something wrong here:
layout.php
PHP:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=windows-1250" />
<title>Edited Rl Map Server 8.6!</title>
<link rel="stylesheet" type="text/css" href="<?php echo $layout_name; ?>/main.css" />
<link rel="stylesheet" type="text/css" href="<?php echo $layout_name; ?>/basic.css" />
<?PHP echo $layout_header; ?>
<script type="text/javascript" src="<?php echo $layout_name; ?>/newsticker.js"></script>
<script type="text/javascript" src="<?PHP echo $layout_name; ?>/jquery.js"></script>
<link rel="shortcut icon" href="<? echo $layout_name; ?>/images/server.ico" type="image/x-icon"/>
<link rel="icon" href="<? echo $layout_name; ?>/images/server.ico" type="image/x-icon"/>
</head>
<body>
<div id="vt_page">
<div id="vt_header">
<div align="center">
<div id="menu">
</div>
</div>
</div>
<div id="vt_container">
<div id="vt_menu">
<div id="vt_news_menu">
<div class="header"><font color="gold">Ogólne</font></div>
<ul>
<li><a href="index.php?subtopic=latestnews">Nowości</a></li>
<li><a href="index.php?subtopic=archive">Archiwum</a></li>
<li><a href="index.php?subtopic=serverinfo"><font color="yellow">Informacje</font></a></li>
<li><a href="/forum"><font color="red">Forum</font></a></li>
<li><a href="index.php?subtopic=faq"><font color="red">FAQ i Poradniki</font></a></li>
</ul>
</div>
<div id="vt_account_menu">
<div class="header"><font color="gold">Konto</font></div>
<ul>
<?php
if($logged)
{
echo '
<li><a href="?subtopic=accountmanagement"><font color="red">Moje konto</font></a></li>
<li><a href="?subtopic=accountmanagement&action=logout">Wyloguj</a></li>';
}
else
{
echo '
<li><a href="?subtopic=accountmanagement">Zaloguj</a></li>
<li><a href="index.php?subtopic=createaccount"><font color="red">Stwórz Konto!</font></a></li>
<li><a href="index.php?subtopic=lostaccount">Odzyskaj Konto</a></li>';
}
?>
<li><a href="index.php?subtopic=tibiarules">Regulamin Serwera</a></li>
<li><a href="index.php?subtopic=sellchar"><font color="yellow">Kup postać</font></a></li>
<?php if($group_id_of_acc_logged >= $config['site']['access_admin_panel']): ?>
<li><a href="index.php?subtopic=adminpanel"><font color="red">Admin Panel</font></a></li>
<?php endif; ?>
<?php if($group_id_of_acc_logged > 0): ?>
<li><a href="index.php?subtopic=namelock"><font color="red">Namelocks</font></a></li>
<?php endif; ?>
</ul>
</div>
<div id="vt_community_menu">
<div class="header"><font color="gold">Społeczność</font></div>
<ul>
<li><a href="index.php?subtopic=whoisonline">Kto jest online?</a></li>
<li><a href="index.php?subtopic=characters">Szukaj Gracza</a></li>
<li><a href="index.php?subtopic=highscores">Statystyki</a></li>
<li><a href="index.php?subtopic=wars"><font color="yellow">Wojny</font></a></li>
<li><a href="index.php?subtopic=guilds">Gildie</a></li>
<li><a href="index.php?subtopic=killstatistics">Ostatnie Zgony</a></li>
<li><a href="index.php?subtopic=top_frags"><font color="red">Top Fraggers</font></a></li>
<li><a href="index.php?subtopic=top_guilds"><font color="red">Top Guilds</font></a></li>
<li><a href="index.php?subtopic=hunt"><font color="yellow">Hunt system</font></a></li>
<li><a href="index.php?subtopic=bans">Zbanowani</a></li>
<li><a href="index.php?subtopic=team">Support</a></li>
</ul>
</div>
<div id="vt_library_menu">
<div class="header"><font color="gold">Biblioteka</font></div>
<ul>
<li><a href="index.php?subtopic=bonusaddon"><font color="lime">Outfit bonus</font></a></li>
<li><a href="index.php?subtopic=houses">Domki</a></li>
<li><a href="index.php?subtopic=galeria">Galeria</a></li>
<li><a href="index.php?subtopic=experiencetable">Tabela Expa</a></li>
</ul>
</div>
<?php if($config['site']['shop_system'] == 1): ?>
<div id="vt_shop_menu">
<div class="header"><font color="gold">Sklep</font></div>
<ul>
<li><a href="index.php?subtopic=buypoints"><font color="red">Kup punkty</font></a></li>
<li><a href="index.php?subtopic=shopsystem"><font color="yellow">Sklep</font></a></li>
<li><a href="index.php?subtopic=wins"><font color="lime">Best Donators</font></a></li>
<?php if($logged): ?>
<li><a href="index.php?subtopic=shopsystem&action=show_history">Historia Tranzakcji</a></li>
<?php endif; ?>
<?php if($group_id_of_acc_logged >= $config['site']['access_admin_panel']): ?>
<li><a href="index.php?subtopic=shopadmin">Shop Admin</a></li>
<?php endif; ?>
</ul>
</div>
<?php endif; ?>
</div>
<div id="vt_content">
<?php echo $main_content; ?>
</div>
<div id="vt_panel">
<div class="top">
<div class="bot">
<div id="vt_panel_module">
<div class="header"><font color="gold"><center>Server Status</center></font></div>
<?PHP
if($config['status']['serverStatus_online'] == 1)
echo '<font color="#00ff00" size="2"><b><center>Soltrion jest - ONLINE</b></font><br><br><font size="2" color="white"><b>Graczy Online:</b></font><font size="2" color="#00ff00"> <div id="players" style="display: inline;">0</div> / 1000 <br><font size="2" color="white"><b>Uptime:</b></font><font size="2" color="#00ff00"> '.$config['status']['serverStatus_uptime'].'</font></font></br></br><font size="2" color="white"><b>IP Servera:</font> <font size="2" color="#00ff00">188.116.52.94<br></b></font></font>';
else
echo '<font color="red" size="2"><b><center>Soltrion jest - OFFLINE</center></b></font><br><br>';
?>
<div class="top">
<div class="bot">
<div id="vt_panel_module">
<div class="header"><font color="gold">Top 10 Players</font></div>
<?PHP
$order = 0;
$skills = $SQL->query('SELECT name,online,level,experience,vocation,promotion FROM players WHERE players.deleted = 0 ORDER BY level DESC, experience DESC LIMIT 10;');
foreach($skills as $skill) {
$order++;
$players_skill .= '<table cellspacing="0" cellpadding="5" border="0" width="100%">
<tr><td width="20%"><font color="#e15f08"><b>'.$order.'.</b></font></td>
<td align="center"><a href="?subtopic=characters&name='.urlencode($skill['name']).'"><font color="red">'.($skill['online']>0 ? "<font color=\"green\">".$skill['name']."</font>" : "".$skill['name']."</font>").'</a></td>
<td align="right"><font color="#e15f08"><b>'.$skill['level'].' lvl</b></font></td>';
}
echo "$players_skill";
?>
</table>
</div>
</div>
</div>
</div>
</div>
</div>
<br>
<div id="vt_panel">
<div class="top">
<div class="bot">
<div id="vt_panel_module">
<div class="header"><font color="gold"><center>Facebook<br><br><iframe src="//www.facebook.com/plugins/like.php?href=https%3A%2F%2Fwww.facebook.com%2Fpages%2FSoltrionzaptoorg%2F354965637889624&send=false&layout=button_count&width=450&show_faces=false&font&colorscheme=light&action=like&height=21" scrolling="no" frameborder="0" style="border:none; overflow:hidden; width:200px; height:21px;" allowTransparency="true"></iframe></center></font></div>
</table>
</div>
</div>
</div>
<br><br>
<script type="text/javascript" src="http://ads.adfreestyle.pl/show/sk0f3338gZ5"></script>
</div>
</div>
</div>
<div id="vt_footer">
<center>Copyrights © 2013 by <b>Soltrion.zapto.org</b> All Rights Reserved.</center>
<a href=""><img src="<?php echo $layout_name; ?>/images/html_valid.png" alt="HTML Valid" /></a>
<a href=""><img src="<?php echo $layout_name; ?>/images/css_valid.png" alt="CSS Valid" /></a>
</div>
</div>
</div>
</body>
</html>
Last edited: