Discovery
Developing myself
Hello guys, this is the first version of the new exercise weapons script!
Feel free to help to make suggestions
If you no have this new sprites, use another.. no have difference (like wooden dummy)
Create the file exercise_training.lua
Added this line in actions.xml
In events.xml
- You will change enable="0" to "1".
In /events/scripts/player.lua
Added this lines in the top of the file
- Go to the function
- Go to the function
Have fun!
Feel free to help to make suggestions

If you no have this new sprites, use another.. no have difference (like wooden dummy)
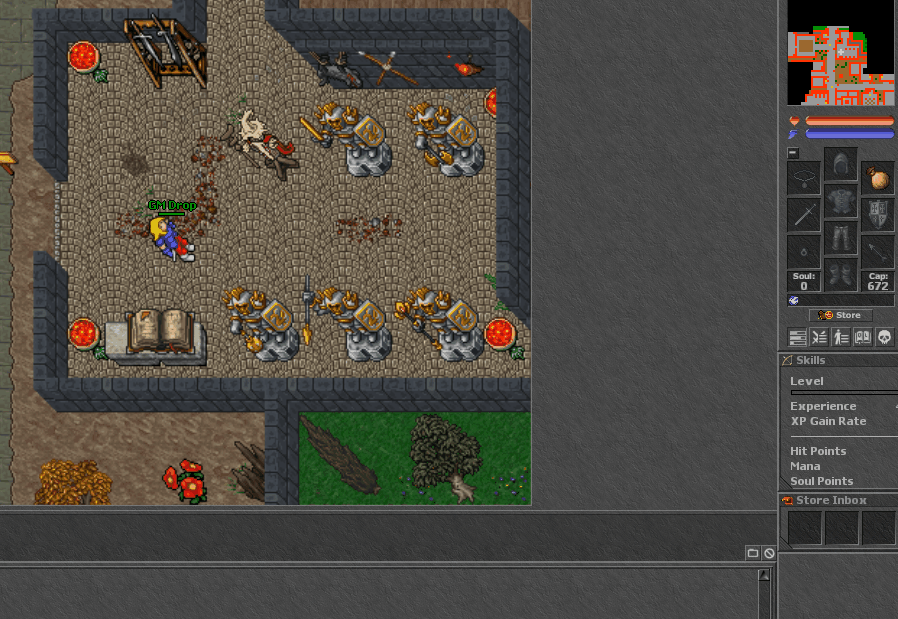
Create the file exercise_training.lua
LUA:
local skills = {
[26397] = {id=SKILL_SWORD,voc=4},
[26398] = {id=SKILL_AXE,voc=4},
[26399] = {id=SKILL_CLUB,voc=4},
[26400] = {id=SKILL_DISTANCE,voc=3,range=CONST_ANI_SIMPLEARROW},
[26401] = {id=SKILL_MAGLEVEL,voc=2,range=CONST_ANI_ENERGY},
[26402] = {id=SKILL_MAGLEVEL,voc=1,range=CONST_ANI_FIRE},
}
------- CONFIG -----//
local dummies = {26403,26404}
local skill_gain = 1 -- per hit
local gain_stamina = 60
local function start_train(pid,start_pos,itemid,fpos)
local player = Player(pid)
if player ~= nil then
local pos_n = player:getPosition()
if start_pos:getDistance(pos_n) == 0 and getTilePzInfo(pos_n) then
if player:getItemCount(itemid) >= 1 then
local exercise = player:getItemById(itemid,true)
if exercise:isItem() then
if exercise:hasAttribute(ITEM_ATTRIBUTE_CHARGES) then
local charges_n = exercise:getAttribute(ITEM_ATTRIBUTE_CHARGES)
if charges_n >= 1 then
exercise:setAttribute(ITEM_ATTRIBUTE_CHARGES, (charges_n-1))
local required = 0
local currently = 0
local voc = player:getVocation()
if skills[itemid].id == SKILL_MAGLEVEL then
required = voc:getRequiredManaSpent(player:getBaseMagicLevel() + 1)/skill_gain
currently = player:getManaSpent()
player:addManaSpent(required - currently)
else
required = voc:getRequiredSkillTries(skills[itemid].id, player:getSkillLevel(skills[itemid].id)+1)/skill_gain
currently = player:getSkillTries(skills[itemid].id)
player:addSkillTries(skills[itemid].id, (required - currently))
end
fpos:sendMagicEffect(CONST_ME_HITAREA)
if skills[itemid].range then
pos_n:sendDistanceEffect(fpos, skills[itemid].range)
end
player:setStamina(player:getStamina() + 60)
if charges_n == 1 then
exercise:remove(1)
return true
end
local training = addEvent(start_train, voc:getAttackSpeed(), pid,start_pos,itemid,fpos)
else
exercise:remove(1)
stopEvent(training)
end
end
end
end
else
stopEvent(training)
end
else
stopEvent(training)
end
return true
end
function onUse(player, item, fromPosition, target, toPosition, isHotkey)
local start_pos = player:getPosition()
if target:isItem() then
if isInArray(dummies,target:getId()) then
if not skills[item.itemid].range and (start_pos:getDistance(target:getPosition()) > 1) then
stopEvent(training)
return false
end
if not player:getVocation():getId() == skills[item.itemid].voc or not player:getVocation():getId() == (skills[item.itemid].voc+4) then
stopEvent(training)
return false
end
player:sendTextMessage(MESSAGE_STATUS_CONSOLE_BLUE, "You started training.")
start_train(player:getId(),start_pos,item.itemid,target:getPosition())
end
end
return true
end
Added this line in actions.xml
XML:
<!-- Training -->
<action fromid="26397" toid="26402" script="exercise_training.lua" allowfaruse="1"/>
In events.xml
- You will change enable="0" to "1".
XML:
<event class="Player" method="onTradeRequest" enabled="1" />
In /events/scripts/player.lua
Added this lines in the top of the file
LUA:
local exercise_ids = {26397,26398,26399,26400,26401,26402}
- Go to the function
function Player::onMoveItem
added this lines in the top.
LUA:
-- Exercise Weapons
if isInArray(exercise_ids,item.itemid) then
self:sendCancelMessage('You cannot move this item outside this container.')
return false
end
- Go to the function
function Player::onTradeRequest
added this lines in the top.
LUA:
if isInArray(exercise_ids,item.itemid) then
return false
end
Have fun!
Last edited by a moderator: