Hi,
I'm trying to figure out how to check if a player has a custom house by name, not any house only a specific one, and if so, how to get the location of that house.
Additionally, I need to create a custom item that only the owner of a custom house can use.
Specifically, I need to be able to check the custom house of a player named Mark for example and I don't know if this can be done using queries or not, and then assign a custom item ID to an item that only Mark can use to teleport to his custom house and nobody else could use it except the house owner.
Does anyone know how I can do this? Any help or examples would be greatly appreciated.
I'm aware that the script linked below works to teleport the player to their house. However, I need to modify it so that it only works for a specific house, and only the house owner can use it.
otland.net
Thanks in advance.
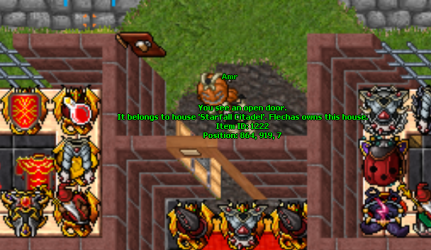
Edit: I had an idea that I think would work. We could use a storage value. If the player bought this item, I could basically assign it to the previous script to make the item work for the player who bought it. However, there is a problem. If another player bought the same item, it would ruin the storage. This would mean that both players would have the same storage.
I'm not sure if this is the best way to write this and I don't know if there is any shortcut function that would catch what I want, but I appreciate any suggestions.
I'm trying to figure out how to check if a player has a custom house by name, not any house only a specific one, and if so, how to get the location of that house.
Additionally, I need to create a custom item that only the owner of a custom house can use.
Specifically, I need to be able to check the custom house of a player named Mark for example and I don't know if this can be done using queries or not, and then assign a custom item ID to an item that only Mark can use to teleport to his custom house and nobody else could use it except the house owner.
Does anyone know how I can do this? Any help or examples would be greatly appreciated.
I'm aware that the script linked below works to teleport the player to their house. However, I need to modify it so that it only works for a specific house, and only the house owner can use it.
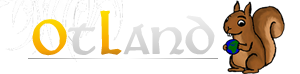
House teleport scroll?
Hello guys, does anyone have a house teleport scroll for tfs 1.3?? like the player uses the scroll, it disapears and he gets teleported to his front door of his house, wich some magic spell? :D
Thanks in advance.
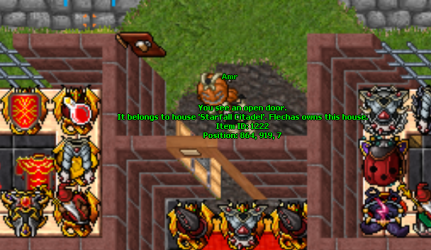
Edit: I had an idea that I think would work. We could use a storage value. If the player bought this item, I could basically assign it to the previous script to make the item work for the player who bought it. However, there is a problem. If another player bought the same item, it would ruin the storage. This would mean that both players would have the same storage.
I'm not sure if this is the best way to write this and I don't know if there is any shortcut function that would catch what I want, but I appreciate any suggestions.
Last edited: