Leo32
Getting back into it...
- Joined
- Sep 21, 2007
- Messages
- 987
- Solutions
- 14
- Reaction score
- 532
EDIT: Skip to the conclusion and Flash Client .DAT breakdown here.
Intro
I've been developing my project for awhile using the flash client:
The client itself is working just fine and suits the project perfectly, but there is an major annoyance with item usability...
The Flash client uses smart-clicks, meaning the client dictates what to do when you right-click on an object:
This data looks to be contained within the appearances.dat file:
https://github.com/Leo32onGIT/TFS-F...a167fb4e3d2fb93d4574fd0559d160140474e3431.dat
Task
The drama is the decoding of the .dat file.
It's spec is different to the standard tibia.dat spec which should be obvious as sprites are now stored as an atlas (?).
Preliminary
The structure of the item blocks/flags looks to be much the same:
C++ Client's Tibia.dat:
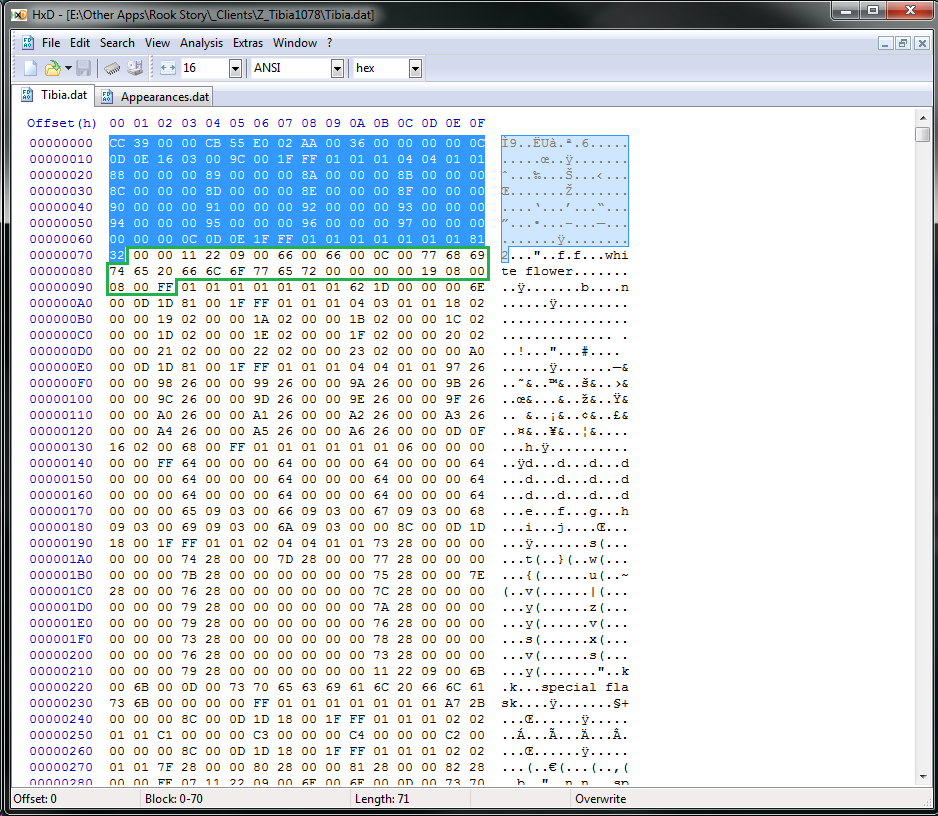
Flash Client's Appearances.dat:

So there shouldn't be any need to reinvent the wheel here.
It's a matter of understanding the structure of the old Tibia.dat file properly, then decipher the new data included in the new Appearances.dat file.
This is my first attempt at trying to reverse engineer a binary file format, so if anyone has any experience or can help in any meaningful way - it would be greatly appreciated.
Intro
I've been developing my project for awhile using the flash client:

The client itself is working just fine and suits the project perfectly, but there is an major annoyance with item usability...
The Flash client uses smart-clicks, meaning the client dictates what to do when you right-click on an object:
- Use
- Use With...
- Look
This data looks to be contained within the appearances.dat file:
https://github.com/Leo32onGIT/TFS-F...a167fb4e3d2fb93d4574fd0559d160140474e3431.dat
Task
The drama is the decoding of the .dat file.
It's spec is different to the standard tibia.dat spec which should be obvious as sprites are now stored as an atlas (?).
Preliminary
The structure of the item blocks/flags looks to be much the same:
C++ Client's Tibia.dat:
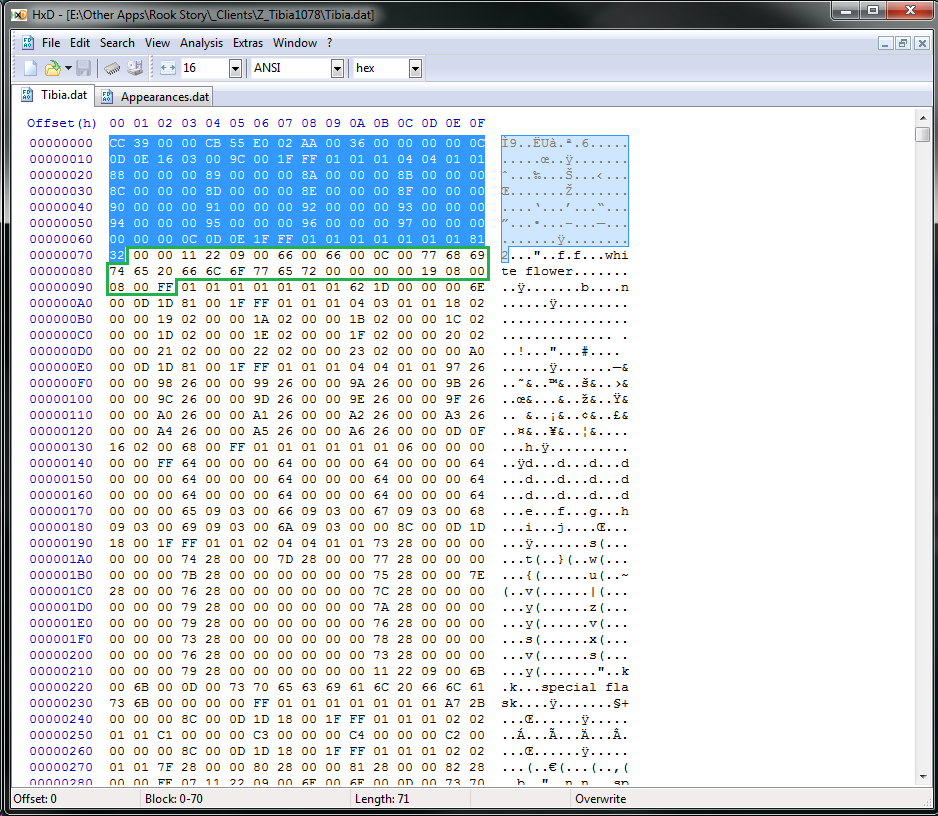
Flash Client's Appearances.dat:

So there shouldn't be any need to reinvent the wheel here.
It's a matter of understanding the structure of the old Tibia.dat file properly, then decipher the new data included in the new Appearances.dat file.
This is my first attempt at trying to reverse engineer a binary file format, so if anyone has any experience or can help in any meaningful way - it would be greatly appreciated.
Last edited: