mar173
Member
- Joined
- Aug 14, 2016
- Messages
- 34
- Reaction score
- 18
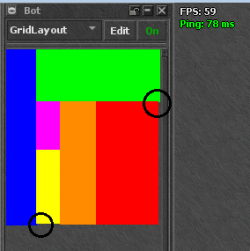
Why does not it stick together? All sides should be equal. Blue Green has more width than Blue Pink Orange Red.
Where is problem? In my code or otclient?
Lua:
local cell_count = 0
local Row
local Grid
local Column
Column = SDK_Class()
function Column:__init(args, parent)
self.rows = {}
self.type = 1
cell_count = cell_count + 1
self.root = SDK_UI:Widget('Panel')
self.root:Set('root_' .. self.type .. '_' .. cell_count, parent)
self.root:SetWidth(args.width)
if args.background_color then
self.root.Widget:setBackgroundColor(args.background_color)
end
end
function Column:AddRow(args)
local row = Row(args, self.root.Widget)
table.insert(self.rows, row)
return row
end
Row = SDK_Class()
function Row:__init(args, parent)
self.columns = {}
self.type = 2
cell_count = cell_count + 1
self.root = SDK_UI:Widget('Panel')
self.root:Set('root_' .. self.type .. '_' .. cell_count, parent)
self.root:SetHeight(args.height)
if args.background_color then
self.root.Widget:setBackgroundColor(args.background_color)
end
end
function Row:AddColumn(args)
local column = Column(args, self.root.Widget)
table.insert(self.columns, column)
return column
end
Grid = SDK_Class()
function Grid:__init(grid)
self.cells = {}
self.type = 3
self.grid = grid
self.root = grid.root
end
function Grid:AddRows(rows)
local prev = false
local total = 0
for i = 1, #rows do
local args = rows[i]
local height = self.root:GetHeight()
if i == #rows then
args.height = height - total
else
args.height = math.floor(args.grid_percent and (height * args.grid_percent) or (height / #rows))
total = total + args.height
end
local cell = self.grid:AddRow(args)
cell.root:SetAnchors({
[modules.corelib.AnchorLeft] = modules.corelib.AnchorLeft,
[modules.corelib.AnchorRight] = modules.corelib.AnchorRight,
--[modules.corelib.AnchorTop] = modules.corelib.AnchorTop,
--[modules.corelib.AnchorBottom] = modules.corelib.AnchorBottom
})
cell.root:SetAnchorsTop(prev and 'prev' or 'parent', prev and modules.corelib.AnchorBottom or modules.corelib.AnchorTop)
if args.cells then
local grid = Grid(cell)
grid:AddCells(args.cells)
self.cells[args.id] = grid
else
self.cells[args.id] = cell
end
prev = true
end
end
function Grid:AddColumns(columns)
local prev = false
local total = 0
for i = 1, #columns do
local args = columns[i]
local width = self.root:GetWidth()
if i == #columns then
args.width = width - total
else
args.width = math.floor(args.grid_percent and (width * args.grid_percent) or (width / #columns))
total = total + args.width
end
local cell = self.grid:AddColumn(args)
cell.root:SetAnchors({
--[modules.corelib.AnchorLeft] = modules.corelib.AnchorLeft,
--[modules.corelib.AnchorRight] = modules.corelib.AnchorRight,
[modules.corelib.AnchorTop] = modules.corelib.AnchorTop,
[modules.corelib.AnchorBottom] = modules.corelib.AnchorBottom
})
cell.root:SetAnchorsLeft(prev and 'prev' or 'parent', prev and modules.corelib.AnchorRight or modules.corelib.AnchorLeft)
if args.cells then
local grid = Grid(cell)
grid:AddCells(args.cells)
self.cells[args.id] = grid
else
self.cells[args.id] = cell
end
prev = true
end
end
function Grid:AddCells(cells)
if self.grid.type == 1 then
self:AddRows(cells)
elseif self.grid.type == 2 then
self:AddColumns(cells)
end
end
local layoutBota = Grid(Row({
height = 200,
}, panel))
layoutBota:AddCells({
{grid_percent = 0.2, id = 'blue', background_color = 'blue'},
{grid_percent = 0.8, id = 'testGrid', cells = {
{grid_percent = 0.3, id = 'green', background_color = 'green'},
{grid_percent = 0.7, id = 'testGrid', cells = {
{grid_percent = 0.2, id = 'testGrid', cells = {
{grid_percent = 0.4, id = 'pink', background_color = 'pink'},
{grid_percent = 0.6, id = 'yellow', background_color = 'yellow'},
}},
{grid_percent = 0.3, id = 'orange', background_color = 'orange'},
{grid_percent = 0.5, id = 'red', background_color = 'red'},
}},
}},
})
SDK_UI:Widget
Lua:
function UI:Widget(type)
local i = {Type = type}
function i:Set(id, parent)
local widget
if parent == nil then
parent = g_ui.getRootWidget()
widget = parent:recursiveGetChildById(id)
else
widget = parent:getChildById(id)
end
if widget == nil then
widget = g_ui.createWidget(self.Type, parent)
widget:setId(id)
end
self.Id = id
self.Widget = widget
self.Parent = parent
end
function i:Show()
self.Widget:show()
end
function i:Raise()
self.Widget:raise()
end
function i:Enable()
self.Widget:enable()
end
function i:SetAnchors(anchors)
for anchorFrom, anchorTo in pairs(anchors) do
self.Widget:addAnchor(anchorFrom, 'parent', anchorTo)
end
end
function i:SetAnchorsLeft(parent, anchor)
self.Widget:addAnchor(modules.corelib.AnchorLeft, parent, anchor)
end
function i:SetAnchorsRight(parent, anchor)
self.Widget:addAnchor(modules.corelib.AnchorRight, parent, anchor)
end
function i:SetAnchorsTop(parent, anchor)
self.Widget:addAnchor(modules.corelib.AnchorTop, parent, anchor)
end
function i:SetAnchorsBottom(parent, anchor)
self.Widget:addAnchor(modules.corelib.AnchorBottom, parent, anchor)
end
function i:Resize(width, height)
self.Widget:resize(width, height)
end
function i:SetWidth(width)
self.Widget:setWidth(width)
end
function i:SetHeight(height)
self.Widget:setHeight(height)
end
function i:GetWidth()
return self.Widget:getWidth()
end
function i:GetHeight()
return self.Widget:getHeight()
end
function i:SetMarginTop(margin)
self.Widget:setMarginTop(margin)
end
function i:SetMarginRight(margin)
self.Widget:setMarginRight(margin)
end
function i:SetMarginBottom(margin)
self.Widget:setMarginBottom(margin)
end
function i:SetMarginLeft(margin)
self.Widget:setMarginLeft(margin)
end
return i
end
Last edited: